iOS Can I inject HTML code in the emails send by mail app?
Solution 1
AFAIK you can't trap emails sent from the built in email client. You can however, write your own app that sends emails with HTML using the MFMailComposeViewController
.
EDIT: Added some example code:
- (void) sendEventInEmail
{
MFMailComposeViewController *picker = [[MFMailComposeViewController alloc] init];
picker.mailComposeDelegate = self;
[picker setSubject:@"Email Subject"];
// Fill out the email body text
NSString *iTunesLink = @"http://itunes.apple.com/gb/app/whats-on-reading/id347859140?mt=8"; // Link to iTune App link
NSString *content = [eventDictionary objectForKey:@"Description"];
NSString *imageURL = [eventDictionary objectForKey:@"Image URL"];
NSString *findOutMoreURL = [eventDictionary objectForKey:@"Link"];
NSString *emailBody = [NSString stringWithFormat:@"<br /> <a href = '%@'> <img src='%@' align=left style='margin:5px' /> </a> <b>%@</b> <br /><br />%@ <br /><br />Sent using <a href = '%@'>What's On Reading</a> for the iPhone.</p>", findOutMoreURL, imageURL, emailSubject, content, iTunesLink];
[picker setMessageBody:emailBody isHTML:YES];
[self presentModalViewController:picker animated:YES];
[picker release];
}
- (void)mailComposeController:(MFMailComposeViewController*)controller didFinishWithResult:(MFMailComposeResult)result error:(NSError*)error
{
// Notifies users about errors associated with the interface
switch (result)
{
case MFMailComposeResultCancelled:
break;
case MFMailComposeResultSaved:
break;
case MFMailComposeResultSent:
break;
case MFMailComposeResultFailed:
break;
default:
break;
}
[self dismissModalViewControllerAnimated:YES];
}
Solution 2
It should look somewhat like this:
// Compose body
NSMutableString *emailBody = [[[NSMutableString alloc] initWithString:@"<html><body>"] autorelease];
[emailBody appendString:@"<p>Hi<br><br>THis is some HTML</p>"];
[emailBody appendString:@"</body></html>"];
// Compose dialog
MFMailComposeViewController *emailDialog = [[MFMailComposeViewController alloc] init];
emailDialog.mailComposeDelegate = self;
[emailDialog setToRecipients:[NSArray arrayWithObject:@"[email protected]"]];
// Set subject
[emailDialog setSubject:@"I got a question!"];
// Set body
[emailDialog setMessageBody:emailBody isHTML:YES];
// Show mail
[self presentModalViewController:emailDialog animated:YES];
[emailDialog release];
As said above, don't forget the delegate 8)
HTH Marcus
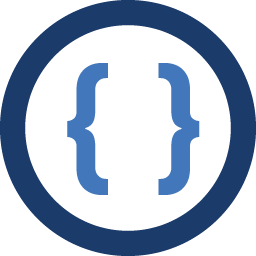
Admin
Updated on August 16, 2022Comments
-
Admin over 1 year
We are running an stationery business and designing email stationeries, signatures etc for people. On PC there's no problem using those because it's easy to add HTML to the email contents in most of the mail clients. However, is this possible with iOS?
How are we doing that now for mobile devices is we tell users to add our SMTP server and send messages through it. How this SMTP works is it's wrapping each email with HTML before sending. I wonder if this can be done directly in iPhone with some kind of post-processing of emails before those are sent?