iPhone: why isn't drawRect getting called?
Solution 1
drawRect: is a method for UIView subclasses. Try to subclass UIView and change the type of the UIView in InterfaceBuilder (see pic).
alt text http://img.skitch.com/20090627-xetretcfubtcj7ujh1yc8165wj.jpg
Solution 2
drawRect
is a method from UIView
and not from UIViewController
, that's why it's not being called.
In your case, it seems that you need to create your custom UIView
and overwrite drawRect
in it, and not in your UIViewController
subclass.
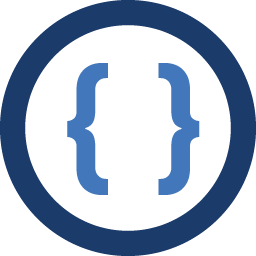
Admin
Updated on September 16, 2022Comments
-
Admin over 1 year
Okay, I guess I'm missing something important, and I can't seem to find the answer. I'm posting all the code, because it's very small.
Can someone please tell me what I'm doing wrong? I've worked on this looking at example after example, for quite a while, and nothing I do seems to work.
When I create the app, I use whichever skeleton gives me a UIViewController. I put a view onto the controller. I create the variables to link into the controller. When I try to connect the UIView in my nib to my UIView, the compiler's okay with it, but it also insists on being connect to "view" as well, or the app crashes. I wouldn't be surprised if that's the issue, but if it is, I can't figure out how to fix it.
Here's the code:
DotsieAppDelegate.h:
#import <UIKit/UIKit.h> @class DotsieViewController; @interface DotsieAppDelegate : NSObject <UIApplicationDelegate> { UIWindow *window; DotsieViewController *viewController; } @property (nonatomic, retain) IBOutlet UIWindow *window; @property (nonatomic, retain) IBOutlet DotsieViewController *viewController; @end
DotsieAppDelegate.m
#import "DotsieAppDelegate.h" #import "DotsieViewController.h" @implementation DotsieAppDelegate @synthesize window; @synthesize viewController; - (void)applicationDidFinishLaunching:(UIApplication *)application { // Override point for customization after app launch [window addSubview:viewController.view]; [window makeKeyAndVisible]; } - (void)dealloc { [viewController release]; [window release]; [super dealloc]; } @end
DotsieViewController.h
#import <UIKit/UIKit.h> @interface DotsieViewController : UIViewController { UIView *dotsieView; } @property (nonatomic, retain) IBOutlet UIView *dotsieView; @end
DotsieViewController.m
#import "DotsieViewController.h" @implementation DotsieViewController @synthesize dotsieView; -(void)drawRect:(CGRect)rect { NSLog(@"here"); CGRect currentRect = CGRectMake(50,50,20,20); UIColor *currentColor = [UIColor redColor]; CGContextRef context = UIGraphicsGetCurrentContext(); CGContextSetLineWidth(context, 2.0); CGContextSetStrokeColorWithColor(context, currentColor.CGColor); CGContextSetFillColorWithColor(context, currentColor.CGColor); CGContextAddEllipseInRect(context, currentRect); CGContextDrawPath(context, kCGPathFillStroke); // [self.view setNeedsDisplay]; } // The designated initializer. Override to perform setup that is required before the view is loaded. - (id)initWithNibName:(NSString *)nibNameOrNil bundle:(NSBundle *)nibBundleOrNil { if (self = [super initWithNibName:nibNameOrNil bundle:nibBundleOrNil]) { // Custom initialization } [self.view setNeedsDisplay]; return self; } - (void)didReceiveMemoryWarning { // Releases the view if it doesn't have a superview. [super didReceiveMemoryWarning]; // Release any cached data, images, etc that aren't in use. } - (void)viewDidUnload { // Release any retained subviews of the main view. // e.g. self.myOutlet = nil; } - (void)dealloc { [super dealloc]; } @end