Is it possible to bind Route Value to a Custom Attribute's Property in ASP.NET Core Web API?
Solution 1
No, it is not possible.
Attribute parameters are restricted to constant values of the following types:
Simple types (bool, byte, char, short, int, long, float, and double)
string
System.Type
enums
object (The argument to an attribute parameter of type object must be a constant value of one of the above types.)
One-dimensional arrays of any of the above types
You cannot nest attributes and you cannot pass non-constant values to attribute parameter. Even though you can declare an attribute parameter as type object, the actual argument you pass in must still be a constant string, bool, etc (declaring it as an object just means you can use any of these types each time you declare the attribute).
Solution 2
Try this Control Panel\All Control Panel Items\Administrative Tools double click SERVICES go to "Wired AutoConfig" right click Properties and set the "Startup Type: " to "Automatic"
Solution 3
One way you can do this is by passing the name of the route parameter to the attribute, and examining the route data in the attribute itself. For example:
[SecureMethod(PermissionRequired = AccessPermissionEnum.DeleteUsers, UserIdsKey = "userIds")]
Then something like:
public AccessPermissionEnum PermissionRequired { get; set; }
public string UserIdsKey { get; set; }
public override void OnActionExecuting(ActionExecutingContext context)
{
// Depending on how you pass your userIds to the action, you
// may need to use something else from context to get the data,
// but it's all available in there.
var userIds = context.ActionArguments[UserIdsKey];
// Do something with the ids.
base.OnActionExecuting(context);
}
Whilst this works, and in certain places it works really well, you should be aware that the filter now has intimate knowledge of the parameter being passed in. For the most part, this usually doesn't matter so much, but it's an approach you shouldn't use everywhere because it will end up adding a lot of coupling if not used well. It's a useful tool to use every now and then.
Related videos on Youtube
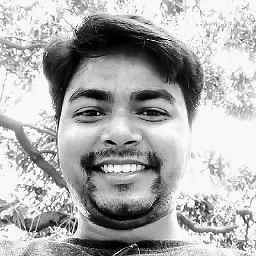
Sunny Sharma
Updated on December 10, 2022Comments
-
Sunny Sharma 11 months
I've a Custom Attribute which I'm using to authorize the request before it hits the Controller Action, in ASP.NET Core Web API. In the image below, "SecureMethod" is the custom attribute.
I want to add a property in the Custom Attribute, which I want to bind to one of the Route Values, please refer to the image below:
I want to bind "userIDs" route-value to one of the Custom Attribute's property ("UserIDs", this is an example attribute).
Is there any way I can bind the route-value to a custom-attribute's property?
TIA
-
Ramhound over 10 yearsWhat version of windows, what browser, what add-ons/extensions are you using. If you load a clean copy of WIndows into a Virtual Machine does this still happen. Does it happen on a Linux installation. More information is required.
-
-
Davidenko over 10 yearsIts not for wireless! That's why your internet's not working.
-
Davidenko over 10 yearsThe Wired AutoConfig (DOT3SVC) service is responsible for performing IEEE 802.1X (And that's not wireless :D ) Just turn it on again ;)
-
Totty.js over 10 yearswhat exactly improves by activating this service?
-
Davidenko over 10 yearsAnd don't stop services if you don't know what they do! Also, after doing that restart your PC and see what happens. Also, maybe somethings wrong with IP adresses but I dont know that, it may be milion reasons.
-
Totty.js over 10 yearswell, thanks.. now was instantly working... but the first time I restarted and I get into my account it was cleaned up, like a new account, same desktop image but no icons, set for high performance and changed the windows colors.. Now I have the stuff back.. so so strange..
-
Davidenko over 10 yearsmaybe virus or something... strange!
-
Totty.js over 10 yearsnever happened since I have win7, maybe the settings changes. Anyway I restated again and was slow again :s
-
Davidenko over 10 years
-
Sunny Sharma over 4 yearsthanks John! there's restriction as not every action will be having this. I need this additional attribute-property set only for certain actions. And so I was hoping to provide this through the Attribute's Property.
-
John H over 4 years@SunnySharma That's fine. It's a property, so it's not requiring a value (the setter is public). It would be a case of seeing if the property does have a value, and then using that to pull something out of the context, otherwise don't get the route value.
-
Sunny Sharma over 4 yearsSure John, I meant "UserIdsKey" variable name is not fixed, for different actions it could be a different parameter, hence I wanted a string based mapping in Custom Attribute Property itself!
-
Edward over 4 years@SunnySharma
UserIdsKey
does not matter, you just need to pass the right parameter name toUserIdsKey
. Andcontext.ActionArguments[UserIdsKey]
it will get your parameter value.