Is it possible to change material design icon color from xml in Android?
Solution 1
For changing icon color try
<ImageButton
android:layout_width="your value"
android:layout_height="your value"
/* and so on ... */
android:tint="YourColor"
/>
Note: the tint color is painted ontop of the image, not a replacement color. So tint #80ff0000
on a black image gives you 50 % red on black, not 50 % red on the background. I.e. this is not equivalent to iOS template images.
Solution 2
You can use the TintImageView
within the appcompat support library and then tinting/coloring the imageview is by simply calling the android:backgroundTint
to tint the imageview into one color.
Xml
<TintImageView
android:layout_width=""
android:layout_height=""
android:src=""
android:backgroundTint="@color/green"/>
or
<ImageView
android:tint="the_color_you_want"/>
Programatically
ImageView yourImageView = findViewById(...)
yourImageView.setColorFilter(Context.getColor(your_color_here))
So the above xml will tint the imageView to color green, means that it will colorize each pixel of the imageview that are visible to green.
Solution 3
I was looking for the same thing but to change it dynamically in the code. I hope this helps someone looking for the same thing.
Create an ImageView for the icon and use setColorFilter on that view.
ImageView imageViewIcon = (ImageView) listItem.findViewById(R.id.imageViewIcon);
imageViewIcon.setColorFilter(getContext().getResources().getColor(R.color.blue));
Solution 4
<vector android:height="48dp" android:viewportHeight="24.0"
android:viewportWidth="24.0" android:width="48dp" xmlns:android="http://schemas.android.com/apk/res/android">
<path android:fillColor="#ca0f0f" android:pathData="M3,5v14c0,1.1 0.89,2 2,2h14c1.1,0 2,-0.9 2,-2V5c0,-1.1 -0.9,-2 -2,-2H5c-1.11,0 -2,0.9 -2,2zm12,4c0,1.66 -1.34,3 -3,3s-3,-1.34 -3,-3 1.34,-3 3,-3 3,1.34 3,3zm-9,8c0,-2 4,-3.1 6,-3.1s6,1.1 6,3.1v1H6v-1z"/>
</vector>
you change color by changing android:fillColor="#ca0f0f"
Solution 5
How to change material icon color in XML
<ImageButton
android:layout_width="YourValue"
android:layout_height="YourValue"
...
android:tint="YourColor" // this line do the magic
/>
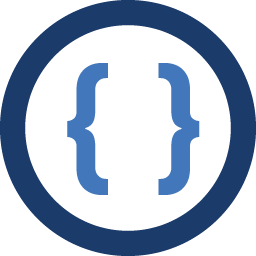
Admin
Updated on January 18, 2020Comments
-
Admin over 4 years
I'm actually trying to use colored icons in my app. I've downloaded the official material design icon pack from here. Now all the icons in this pack are either white, grey or black. But I want the icons to be of a different color. Something like the icons on the left side in this image. The phone phone and mail icons are blue. How can I accomplish this?