Is it possible to configure CLion to compile source files in a project independently?
Solution 1
You could define multiple executables in the CMakeLists.txt for each problem.
instead of
add_executable(projecteuler ${SOURCE_FILES})
you could define
add_executable(problem1 problem1.c)
add_executable(problem2 problem2.c)
Then you get for each executable (problem1, problem2 etc.) a run configuration, which you can run independently. In this case you won't have to rewrite every time, instead you just add the new source file to a new executable.
Solution 2
Even I was facing the same problem, it's a tiring job to edit CMake file and add executable every time. So I found a solution to this,
There is a plugin which does it seamlessly for you.
Just add this plugin to your CLion and whichever file you want to make is executable right click and add it as Executable,
It will automatically edit your CMake file.
Link:
https://plugins.jetbrains.com/plugin/8352-c-c--single-file-execution
Solution 3
You can use
cmake_minimum_required(VERSION 2.8.4)
add_subdirectory(src/prj1)
add_subdirectory(src/prj2)
then in each directory create an other CMakeLists.txt like this one :
cmake_minimum_required(VERSION 2.8.4)
project(prj1)
set(EXEC_NAME prj1)
set(SOURCE_FILES
main_prj1.cpp
x.cpp
y.cpp
)
set(CMAKE_CXX_FLAGS "${CMAKE_CXX_FLAGS} -std=c++11")
set(EXECUTABLE_OUTPUT_PATH ../../dist/${CMAKE_BUILD_TYPE})
add_executable(${EXEC_NAME} ${SOURCE_FILES})
You can use file(GLOB SOURCE_FILES *.cpp)
if you want to automatically add files in your compilation. But keep in mind that this "trick" is strongly not encouraged.
This will also automatically add build configurations to CLion.
Solution 4
You can add these lines to your CMakeLists.txt .
#GLOB_RECURSE will find files in all subdirectories that match the globbing expressions and store it into the variable.
file(GLOB_RECURSE APP_SOURCES *.c)
foreach (testsourcefile ${APP_SOURCES})
#get filename without extension
get_filename_component(testname ${testsourcefile} NAME_WE)
#add executable for all file
add_executable(${testname} ${testsourcefile})
endforeach (testsourcefile ${APP_SOURCES})
You will have to reload cmake project (Tools -> Cmake) every time you add or delete files from your project. Also build time will increase if you add more number of files. Alternative you can create more directories and subdirectories and use file(GLOB_RECURSE APP_SOURCES path/to/*.c)
so it will build only those files.
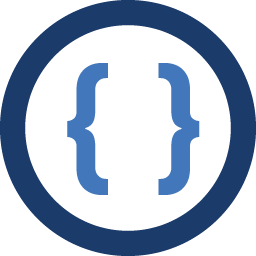
Admin
Updated on June 25, 2022Comments
-
Admin about 2 years
I am currently doing some Project Euler challenges in C using the JetBrains CLion IDE. When I completed these in Python and Java (in PyCharm and IntelliJ, respectively), I was always able to create a single project named "ProjectEuler" and add any number of source files that I could execute independently. However, it seems the same isn't possible in CLion. Each of the source files I use to solve a problem contains a main() function, and CMake is unhappy about that everytime I attempt to compile ("multiple definition of 'main'").
Is there a way to configure CLion to only compile and execute a single source file in a project at a time without having to rewrite my CMakeLists.txt or create a new project for every problem everytime?
I'm aware that C is compiled and not interpreted like Java or Python, but I could conceivably just compile every single source file manually. Is there a way to configure CLion to do the same? If so, how?
-
yotamN about 9 yearsThere isn't a way to make CLion add the source automatically? I know it's not much to add it but it's could be better
-
ipa about 9 yearsIf you add a new C++ source or header file you can check "Add to targets" and then select the targets/executables where you want to add the file in the CMakeLists.txt. But the target has to be already in the CMakeLists.txt file.
-
corochann about 8 years@InvisibleUn1corn I agree that it's nice if we can add
add_executable
more easily. For this purpose, I made a plugin for CLion "C/C++ Single File Execution Plugin".