Is it possible to update a url link based on user text input?
Solution 1
Here's one in plain JS that updates as you type:
<a id="reflectedlink" href="http://www.google.com/search">http://www.google.com/search</a>
<input id="searchterm"/>
<script type="text/javascript">
var link= document.getElementById('reflectedlink');
var input= document.getElementById('searchterm');
input.onchange=input.onkeyup= function() {
link.search= '?q='+encodeURIComponent(input.value);
link.firstChild.data= link.href;
};
</script>
Note:
no inline event handler attributes (they are best avoided);
assigning both keyup and change, to try to get keyboard updates as they happen and ensure that all other updates get caught eventually;
the use of
encodeURIComponent()
, necessary in case the search term has any non-ASCII or URL-special characters in;setting the
search
property of a Location (link) object to avoid having to write out the whole URL again;setting the
data
of the Text node inside the link to reflect the full URL afterwards. Don't setinnerHTML
from user input as it may have HTML-special characters like&
and<
in.
Solution 2
#1: you need some forms
#2: you need to catch when the form is submitted
#3: based on the form's submission change the url
Here is a demo: http://jsfiddle.net/maniator/K3D2v/show/
here is the code: http://jsfiddle.net/maniator/K3D2v/embedded/
HTML:
<form id="theForm">
<input id='subj'/>
<input type='submit'/>
</form>
JS:
var theForm = document.getElementById('theForm');
var theInput = document.getElementById('subj');
theForm.onsubmit = function(e){
location = "http://jsfiddle.net/maniator/K3D2v/show/#"
+ encodeURIComponent(theInput.value);
return false;
}
Solution 3
This is the solution I deviced in a matter of seconds:
<input type="text" name="prog_site" id="prog_site" value="http://www.edit-me.com" />
<a href="#" onclick="this.href=document.getElementById('prog_site').value" target="_blank">Open URL</a>
No complex javascript, no extra quotes nor functions required. Simply edit the ID tag to your needs and it works perfectly.
Solution 4
I'd suggest using a cross browser library such as jQuery rather than straight JavaScript. With jQuery, you'd add a click handler for your button, grab the value of the input, build your URL, and set window.location to go to the new url
HTML
<input type="text" id="q" />
<input type="button" id="submit" value="submit" />
JavaScript
$(function () {
$('#submit').click(function() {
var url = "http://www.google.com/search?q=";
url += $('#q').val();
window.location = url;
});
});
Solution 5
You could try this;
<script type="text/javascript">
function changeText2(){
var userInput = document.getElementById('userInput').value;
var lnk = document.getElementById('lnk');
lnk.href = "http://www.google.com?q=" + userInput;
lnk.innerHTML = lnk.href;
}
</script>
Here is a link : <a href="" id=lnk>nothing here yet</a> <br>
<input type='text' id='userInput' value='Enter Search String Here' />
<input type='button' onclick='changeText2()' value='Change Link'/>
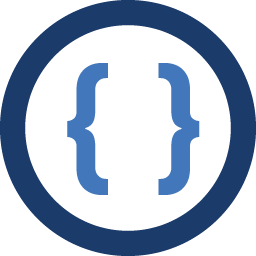
Admin
Updated on July 27, 2020Comments
-
Admin almost 4 years
For instance, I know that it is possible to do something in Javascript that allows users to update text based on user text input:
<script type="text/javascript"> function changeText2(){ var userInput = document.getElementById('userInput').value; document.getElementById('boldStuff2').innerHTML = userInput; } </script> <p>Welcome to the site <b id='boldStuff2'>dude</b> </p> <input type='text' id='userInput' value='Enter Text Here' /> <input type='button' onclick='changeText2()' value='Change Text'/>
View the above code in action at: tizag.com/javascriptT/javascript-innerHTML.php
However, instead of the above code, I would like to know if it's possible to do something similar for a url link. Below I've placed a step by step example of what I would like to happen upon the user inputing text:
Original Link: http://www.google.com/search?q=
User Input in Text Field: espn
User clicks button to submit text in text field
Final Link: http://www.google.com/search?q=espn
Thanks for your help...BTW...if you can't tell I'm a bit of a novice so detail is appreciated.
-
Loktar almost 13 yearsI have to agree with @Neal on this one, question wasn't even tagged jQuery.
-
Naftali almost 13 years@Craig -- also try to use form.submit not input.click ... or else the form will be submitted.
-
Loktar almost 13 yearsoh ho ho, he scores with the input.onchange=input.onkeyup
-
Naftali almost 13 years@bobince - a good 1-up on your part ^_^. I did not add the onkeyup to my answer, but that was a good add on :-D
-
Craig M almost 13 yearsYou don't need a form. It adds nothing but extra bandwidth.
-
Craig M almost 13 yearsThere's a very good reason to use jQuery. As his application grows, he'll undoubtedly get to the point where he needs to write some code that performs differently cross browser. Start with jQuery (or Zepto if jQuery is too big for your taste) and you don't have a mishmash of native DOM manipulation code mixed in with jQuery code. There's no reason to create a form as in your example. You've just created excessive markup.
-
Raynos almost 13 years@CraigM You need a form if you want server-side support.
-
Hcabnettek almost 13 yearsI don't feel jQuery is a bad solution here. It's easy to use for newbies, as this guy likely is, and it's cross browser compatible. Basically substitute any modern dom manipulating library and do something similar. I don't see any need to submit the value unless they want to store that data. Therefore I don't really see a need for a form or submit button here. A simple button and some script should accomplish the task/
-
Craig M almost 13 yearsThere's no server side component to this question, hence you don't need the form.
-
Naftali almost 13 years@CraigM -- and if the user has javascript disabled -- *poof* the form does not work anymore (when there is no
form
tags)... -
Craig M almost 13 yearsThen you can't update the href of a link dynamically which is what he wants to do. Are you really going to submit back to the server and rerender an entire page to update a single link? I don't think so. If you do, you're making very bad design choices.
-
Amit Patil almost 13 yearsBad design choices? No, you're being commendably conscientious. Progressive enhancement demands you make it work without script first, then smooth it up with client-side niceness afterwards. Whether it makes sense practically to do so in this case, or whether it's excessive/pointless, depends on what the ultimate aim of the code is... not enough information in the question to guess that bit!
-
Blake Russo about 7 yearsYou rock man +1 for exactly what I was looking to do. I was trying to do the same only not for google but a custom search for other similar OpenSearch formatted URLS. I checked the reflected link URL to mine, then updated the link.search= to be more then the ?=. I was trying to mimic the behavior of "search engines" in Google Chrome Browser on a website. Thanks!
-
dimitrieh almost 4 years@bobince Thanks for this example! How can I make it so that there is no
?
injected by the code? For example, I just want to add/10
to the end of a URL, not?/10