Is there a difference between int *x and int* x in C++?
Solution 1
The famous Bjarne Stroustrup, notable for the creation and the development of the C++, said ...
The choice between "int* p;" and "int *p;" is not about right and wrong, but about style and emphasis. C emphasized expressions; declarations were often considered little more than a necessary evil. C++, on the other hand, has a heavy emphasis on types.
A "typical C programmer" writes "int *p;" and explains it "*p is what is the int" emphasizing syntax, and may point to the C (and C++) declaration grammar to argue for the correctness of the style. Indeed, the * binds to the name p in the grammar.
A "typical C++ programmer" writes "int* p;" and explains it "p is a pointer to an int" emphasizing type. Indeed the type of p is int*. I clearly prefer that emphasis and see it as important for using the more advanced parts of C++ well.
So, there's no difference. It is just a code style.
From here
Solution 2
To the compiler, they have exactly the same meaning.
Stylistically, there are arguments for and against both.
One argument is that the first version is preferable because the second version:
int* x, y, z;
implies that x
, y
and z
are all pointers, which they are not (only x
is).
The first version does not have this problem:
int *x, y, z;
In other words, since the *
binds to the variable name and not the type, it makes sense to place it right next to the variable name.
The counter-argument is that one shouldn't mix pointer and non-pointer type in the same declaration. If you don't, the above argument doesn't apply and it makes sense to place the *
right next to int
because it's part of the type.
Whichever school of thought you decide to subscribe to, you'll encounter both styles in the wild, and more (some people write int * x
).
Solution 3
No difference at all.
It is just a matter of style.
I personally prefer this:
int *x;
over this,
int* x;
Because the latter is less readable when you declare many variables on the same line. For example, see this:
int* x, y, z;
Here x
is a pointer to int
, but are y
and z
pointers too? It looks like they're pointers, but they are not.
Solution 4
There is no difference. I use the int* x
form because I prefer to keep all of the type grouped together away from the name, but that kind of falls apart with more complex types like int (*x)[10]
.
Some people prefer int *x
because you can read it as "dereferencing x
gives you an int
". But even that falls apart when you start to use reference types; int &x
does not mean that taking the address of x
will give you an int
.
Another reason that you might prefer int *x
is because, in terms of the grammar, the int
is the declaration specifier sequence and the *x
is the declarator. They are two separate parts of the declaration. This becomes more obvious when you have multiple declarators like int *x, y;
. It might be easier to see that y
is not a pointer in this case.
However, many people, like myself, prefer not to declare multiple variables in a single declaration; there isn't exactly much need to in C++. That might be another reason for preferring the int* x
form.
There's only one rule: be consistent.
Solution 5
To the compiler they are the same
But at the same time the difference is readabilty when there are multiple variables
int *x,y
However this is more misleading
int* x,y
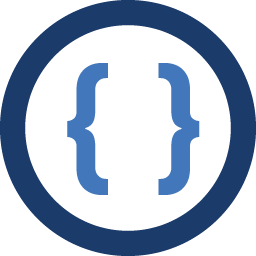
Admin
Updated on April 11, 2020Comments
-
Admin about 4 years
I'm getting back into my C++ studies, and really trying to understand the basics. Pointers have always given me trouble, and I want to make sure I really get it before I carry on and get confused down the road.
Sadly, I've been stymied at the very outset by an inconsistency in the tutorials I'm reading. Some do pointer declarations this way:
int *x
and some do it this way:
int* x
Now the former of the two seems to be by far the most common. Which is upsetting, because the second makes much more sense to me. When I read int *x, I read "Here is an int, and its name is *x", which isn't quite right. But when I read the second, I see "here is an int pointer, and its name is x", which is pretty accurate.
So, before I build my own mental map, is there a functional difference between the two? Am I going to be a leper outcast if I do it the second way?
Thanks.
-
Jason Sperske about 11 yearsAnd personally
int *x
is more intuitive. -
cdhowie about 11 yearsThat's not the reason we prefer
int *x
, we prefer it because it's less error-prone when you are declaring multiple variables on the same line. Prefer forms that are error-resistant. -
cdhowie about 11 yearsAnd, unfortunately, Stroustrup is wrong.
-
Grijesh Chauhan about 11 yearsgood answer Nawaz long time... read my comment to OP question...
-
Joseph Mansfield about 11 years@cdhowie Fair point. I added a paragraph on that too!
-
Thanakron Tandavas about 11 years@cdhowie Why do you think Stroustrup is wrong ?
-
Praetorian about 11 yearsI agree with you completely, but I'd also recommend a good ass kicking for anyone who writes either of those 2 lines in real code.
-
Joseph Mansfield about 11 years@cdhowie In what way? I haven't seen a study into the amount of C++ programmers that do it either way. I feel like I see
int* p
more often than I doint *p
, and Stroustrup clearly thinks he does too. -
cdhowie about 11 yearsBecause Stroustrup prefers a form that is prone to readability errors.
int* x, y;
You will have to spend time trying to figure out what the programmer intended -- did he meany
to be a pointer or not? All we know is:y
is not a pointer, and the programmer is incompetent because he did not make his intent clear. We don't know ify
was supposed to be a pointer, and we shouldn't be wasting time trying to figure out what the programmer meant. Theint* x
form is not error-resistant. -
Admin about 11 yearsThe readability errors arise from declaring more than one variable on a single line. There's no good reason to do so, and it just causes more potential problems than it solves (which is laziness, basically) and it's why most decent coding standards you'll meet when working for real companies disallow it.
-
James Kanze about 11 years@cdhowie But who writes things like
int* x, y;
? In general, coding guidelines insist that you don't put two declarations on the same line. Such things did occur in old C, when you had to declare all of your variables at the top of the block. For a nested loop, for example, you might writeint i, j, k;
. In C++, of course (and modern C), each of these declarations would be in thefor
statement. -
James Kanze about 11 yearsBut no reasonable programmer would write more than one declaration on a line anyway.
-
Joseph Mansfield about 11 years@cdhowie I think he'd also say that declaring multiple variables in one declaration was what "typical C programmers" do.
-
James Kanze about 11 years@cdhowie But who declares multiple variables on the same line. That's a misfeature, left over from C, which all good programmers avoid.
-
James Kanze about 11 years@sftrabbit In the early days of C, when you had to declare all of your variables at the top of the block, there were contexts where it could be justified. In C++, never.
-
Admin about 11 yearsUnfortunately,
int* x, y;
meansy
is anint
, not anint*
. -
Grijesh Chauhan about 11 years@JamesKanze that is why this question may rise :) Nawaz's suggestion is correct/good as my experience (I posted to question.)
-
Nawaz about 11 years@JamesKanze: What is unreasonable about this declaration
point x, y, z;
? -
James Kanze about 11 years@Nawaz What's reasonable about it? Putting it on three lines would be far more readable. (But I'd want to see the context. I'm willing to admit that there might be exceptions. But only when the three types are identical, as when we'd declare
int i, j, k'
in C, before we could put the declarations directly in thefor
.) -
Nawaz about 11 years@JamesKanze: Well the only exception I see when you declare pointer and non-pointer on the same line. But that is trap, as it so easy to fall prey into.
-
Lightness Races in Orbit about 11 yearsNo, the counter-argument is that this argument is ridiculous. How often do you write
int* x, y
, really? There are far more important concerns at work here. :) -
Lightness Races in Orbit about 11 years@cdhowie: Don't be ridiculous. How often do you write
int* x, y
, really? There are far more important concerns at work here. -
Lightness Races in Orbit about 11 years*yawn* This again. It's an edge case, and there are far more important concerns at work here.
-
NPE about 11 years@LightnessRacesinOrbit: Pros and cons aside, I personally think there are more important issues to argue about than where to place the whitespace ;-)
-
Lightness Races in Orbit about 11 years@NPE: That is also true. But within the scope of arguing about the whitespace... :P
-
Lightness Races in Orbit about 11 yearsI enjoy how @cdhowie appears to speak for an entire 50% of the programmer population without even consulting them first!
-
cdhowie about 11 years@LightnessRacesinOrbit Within the context of the original answer posed here, "we" referred to the same people that sftrabbit referred to in the statement "some people prefer..." In this context, "we" refers to "those of us who do prefer this form do so because..." I never implied that I spoke for 50% of the programmer population, and if you inferred that meaning from my statement then you misread it.
-
Lightness Races in Orbit about 11 years@cdhowie: Nothing that you just said changes the fact that you took it upon yourself to speak for everybody "who do prefer this form".
-
cdhowie about 11 years@LightnessRacesinOrbit Very well. I shall therefore amend this statement to have "we" refer to "the many I have encountered during my career who prefer this form."
-
Lightness Races in Orbit about 11 years@cdhowie: Thank you :) Anyway,
int *x
might be error-resistant in that one rare edge case, but it's certainly not misconception-resistant in the way thatint* x
is. -
cdhowie about 11 years@LightnessRacesinOrbit I disagree with the linked rant. Issues of compiler grammar and/or newbie confusion about the way the language works are not important to me. What's important to me is maintainability of code, and the most important factor in maintainability is the ability to correctly and with a minimum of confusion infer the original programmer's intent. Only form 3 (in the context of a multi-variable declaration, which admittedly are rare, but I still run across them from time to time) conveys the original programmer's intent with 100% clarity.
-
Lightness Races in Orbit about 11 years@cdhowie I'm sorry that you don't care about our industry.
-
cdhowie about 11 years@LightnessRacesinOrbit Of course I do -- I prefer forms that make my job easier. The real problem here is not the debate between which form to use, the problem is that the language itself is flawed in this respect. It would have been better to have pointer and array specifiers be on the type (
int* x, y;
meaning what it looks like andchar[10] x, y;
declaring two arrays). Code should be written to be maintainable, and hence I prefer forms that further that goal. I would rather see newbies writing maintainable, less confusing code. -
Lightness Races in Orbit about 11 years@cdhowie: Me too, which is why newbies under my tutelage shall write the type as the type is.
int*
is the type. That's non-confusing, and maintainable. Thanks -
James Kanze about 11 years@Nawaz But I can't see any reasonable case where you would declare both a pointer and a non-pointer in the same statement.