Is there a way to enable controls in video player playing youtube videos flutter web
Solution 1
So what I finally did was a very simple technique. I imported the chewie video player and opened its files from the dart plugins inside the external libraries. Then I changed all the video_player/video_player.dart to ext_video_player/ext_video_player.dart. This worked as ext_video_player is just an extension of video player
Warning :- This will affect all your projects using chewie player. Make this change, build the project, and then remove the changes
So what you do is go to the external libraries folder, then open Dart Packages, and scroll down to the folder chewie-0.12.0 (Or whatever package you are using)\
Then open the source folder, and go to these files :-\
- chewie_player.dart
- cupertino_controls.dart
- cupertino_progress_bar.dart
- material_controls.dart
- material_progress_bar.dart
- player_with_controls.dart\
Now in all these files, you have to scroll to the top and find this line
import 'package:video_player/video_player.dart';
And replace it with
import 'package:ext_video_player/ext_video_player.dart';
Do remember to import the ext_video_player package in the pubspec.yaml. Read the instruction for this package but basically you can provide a youtube url is the
VideoPlayer.network()
thing. I hope this solves your problem. You can also use web-views for apps or iframes for website if you wish though its not recommended
Solution 2
Since the Dart Packages will be deleted when running flutter clean
, using @Siddharth answer, I fork Chewie and edit according to @Siddharth answer (change video_player to ext_video_player).
You can change your Chewie to my git in the pubspec.yaml:
chewie:
git:
url: https://github.com/azhar-rafiq/chewie/
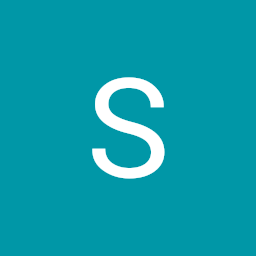
Siddharth Agrawal
Updated on December 23, 2022Comments
-
Siddharth Agrawal over 1 year
I am making a page to show a lot of videos from youtube and am using the ext_video_player in flutter web. But now I want to enable show controls. I also want it to be possible for an app. I saw in this stack over flow post. But the chewie player says that it needs a video controller player from video_player not ext_video_player. Is there any other way to do this so that it works both for flutter web and app and also allows youtube videos. the youtube player plugin is not a choice because of some reasons.I have been unable to make it for even one. Code using rn
class videoBox2 extends StatefulWidget { String Video; videoBox2(this.Video); @override _videoBox2State createState() => _videoBox2State(Video); } class _videoBox2State extends State<videoBox2> { String Video; bool error = false; _videoBox2State(this.Video); VideoPlayerController _controller; @override void dispose(){ super.dispose(); _controller.dispose(); } @override void initState(){ super.initState(); _controller = VideoPlayerController.network( Video, ); _controller.initialize().then((value) { setState(() { }); }); _controller.addListener(() { if (_controller.value.hasError) { setState(() { error = true; print(Video); }); } }); } @override Widget build(BuildContext context) { return error?Container( decoration: BoxDecoration( color: Colors.white, borderRadius: BorderRadius.all(Radius.circular(15)) ), child: Image(fit:BoxFit.cover,image:NetworkImage("https://hiapseng-thailand.com/wp-content/themes/skywalker/facilities/video-placeholder.jpg")) ):GestureDetector( onTap:(){ _controller.value.isPlaying? _controller.pause() :_controller.play(); }, child: Container( width:MediaQuery.of(context).size.width*0.7, decoration: BoxDecoration( color: Colors.white, borderRadius: BorderRadius.all(Radius.circular(15)) ), child: VideoPlayer(_controller), ) ); } }