Is there a way to iterate through lua dictionary in order that it's created?
12,867
EDIT: Changed the answer, the old one is below for reference
-- function definition
function addNewItem(keyTable, myTable, key, value)
table.insert(keyTable, key)
myTable[key] = value
end
To add a new pair into the table:
-- you may need to reset keyTable and myTable before using them
keyTable = { }
myTable = { }
-- to add a new item
addNewItem(keyTable, myTable, "key", "value")
Then, to iterate in the order the keys were added:
for _, k in ipairs(keyTable) do
print(k, myTable[k])
end
OLD ANSWER
Are you the one creating the table (Lua calls these tables and not dictionaries)?? If so, you could try something like the following:
-- tmp is a secondary table
function addNew(A, B, key, value)
table.insert(A, key)
B[key] = value
end
-- then, to browse the pairs
for _,key in ipairs(table) do
print(key, B[key])
done
The idea is that you use two tables. One holds the 'keys' you add (A) and the other (B) the actual values. They look like this:
Since A pairs the keys in a manner like
1 - key1
2 - key2
...
Then ipairs(A) will always return the keys in the order you added them. Then use these keys to access the data
data = B[key1]
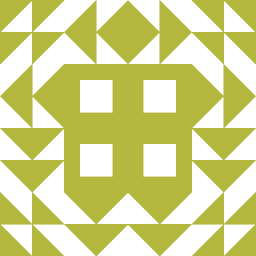
Author by
Alex Smoke
Updated on June 04, 2022Comments
-
Alex Smoke 12 months
I need to iterate through Lua dictionary in the order that it's created.
For example:
t = { ['some'] = 'xxx', ['random'] = 'xxx', ['data'] = 'xxx', ['in'] = 'xxx', ['table'] = 'xxx', }
Normal iterating with pairs gives a random sequence order:
for key, val in pairs(t) do print(key.." : "..val) end random : xxx some : xxx data : xxx table : xxx in : xxx
I need:
some : xxx random : xxx data : xxx in : xxx table : xxx
-
Alex Smoke over 7 yearsSorry, that's not clear enough for me. I already have a table created and just need to iterate through it. Can you please explain more? How to call your addNew() function on my table "t" ?
-
neoaggelos over 7 yearsDo you create the table, by adding the 'key-value' pairs by hand? Or do you use something like 'myTable = createATableFromRandomData(data)'??
-
Alex Smoke over 7 yearsJust by hand, it's stored in a separate file and I need to iterate through it.
-
neoaggelos over 7 yearsIve updated the answer, see if that helps
-
Alex Smoke over 7 yearsThat works well, thank you. Not exactly how I was expecting, because there will be a lot of additional code - my tables are pretty big and with this method they will be twice bigger (tables are generated from third-party app, so there is no chance to change their structure). But thank you anyway, your method works.
-
neoaggelos over 7 yearsI'm glad I helped. Same thing could be done with metatables as well, although it's quite advanced and not worth the fuss. Additionally, myTable and keyTable could actually be the same table, no reason to separate them whatsoever.