Is there a way to make a checkbox list in flutter where the user can edit the label of that checkbox? For example a task that needs to be completed
Solution 1
You just need to return a ListTile
in your instead of Text
inside your itemBuilder
. The ListTile
will have a CheckBox
as a leading
(takes a widget that will appear on the left side), Text
as a title
, and at last, an IconButton
as a trailing
(takes a widget that will appear on the right side). When cliked an AlertDialog
that has a TextField
appears to edit the text editing the text (title
).
List<String> listItems = [];
List<bool> listCheck = [];
final TextEditingController eCtrl = new TextEditingController();
final TextEditingController _textFieldController =
new TextEditingController();
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: new AppBar(
title: new Text("Dynamic Demo"),
),
body: new Column(
children: <Widget>[
new TextField(
controller: eCtrl,
onSubmitted: (text) {
setState(() {
listItems.insert(0, text);
listCheck.add(false);
eCtrl.clear();
});
},
),
new Expanded(
child: new ListView.builder(
itemCount: listItems.length,
itemBuilder: (BuildContext context, int index) {
return new ListTile(
leading: Checkbox(
value: listCheck[index],
onChanged: (value) {
setState(() {
listCheck[index] = !listCheck[index];
});
},
),
title: Text(listItems[index]),
trailing: IconButton(
icon: Icon(Icons.edit),
onPressed: () {
showDialog(
context: context,
builder: (context) {
return AlertDialog(
title: Text('TextField in Dialog'),
content: TextField(
controller: _textFieldController,
decoration: InputDecoration(
hintText: "${listItems[index]}"),
),
actions: <Widget>[
new FlatButton(
child: new Text('Done'),
onPressed: () {
setState(() {
listItems[index] =
_textFieldController.text;
_textFieldController.clear();
});
Navigator.of(context).pop();
},
),
],
);
},
);
},
),
);
},
),
),
],
),
);
You only have to add the CheckBox
functionality.
Solution 2
You can achieve this with DataTable
widget. It is easy to use and can have editable rows. Adding check list to them is easy as well. You can sort them too. Refer to this tutorial. Do as the tutorial say and you will have a list with checking and deleting ability. But to be able to edit the rows, you need to add this line of code to your DataCells in DataRow:
showEditIcon: true,
. I think the tutorial is great with great explanation. But if you need more help, I'll be glad to help.
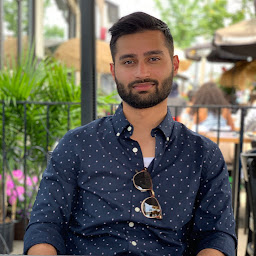
Asher Haque
Updated on December 15, 2022Comments
-
Asher Haque over 1 year
I am trying to make a UI for an app that lets the user list tasks and check them off as they get them done. But I want the user to be able to seamlessly edit the names of those tasks and then hit a checkbox for completion.
I have tried using a ListView.Builder from this tutorial
https://miro.medium.com/max/186/1*gE4Qj0fFgLFGlSUiy-rn2w.gif (you might have to copy and paste the Link)
It's just I want to be able to have a checklist show up
Reference:https://medium.com/@DakshHub/flutter-displaying-dynamic-contents-using-listview-builder-f2cedb1a19fb
class DyanmicList extends State<ListDisplay> { List<String> litems = []; final TextEditingController eCtrl = new TextEditingController(); @override Widget build (BuildContext ctxt) { return new Scaffold( appBar: new AppBar(title: new Text("Dynamic Demo"),), body: new Column( children: <Widget>[ new TextField( controller: eCtrl, onSubmitted: (text) { litems.add(text); eCtrl.clear(); setState(() {}); }, ), new Expanded( child: new ListView.builder ( itemCount: litems.length, itemBuilder: (BuildContext ctxt, int Index) { return new Text(litems[Index]); } ) ) ], ) ); } }
-
Asher Haque over 4 yearsThank you so much for your reply I will try DataTable widget right now, but I don't see the link for the tutorial you mentioned