Is there a way to run a command line command from JScript (not javascript) in Windows Scripting Host (WSH) cscript.exe?
Solution 1
You can execute DOS commands using the WshShell.Run
method:
var oShell = WScript.CreateObject("WScript.Shell");
oShell.Run("timeout /t 10", 1 /* SW_SHOWNORMAL */, true /* bWaitOnReturn */);
If you specifically need to pause the script execution until a key is pressed or a timeout elapsed, you could accomplish this using the WshShell.Popup
method (a dialog box with a timeout option):
var oShell = WScript.CreateObject("WScript.Shell");
oShell.Popup("Click OK to continue.", 10);
However, this method displays a message box when running under cscript as well.
Another possible approach is described in this article: How Can I Pause a Script and Then Resume It When a User Presses a Key on the Keyboard? In short, you can use the WScript.StdIn
property to read directly from input stream and this way wait for input. However, reading from the input stream doesn't support timeout and only returns upon the ENTER
key press (not any key). Anyway, here's an example, just in case:
WScript.Echo("Press the ENTER key to continue...");
while (! WScript.StdIn.AtEndOfLine) {
WScript.StdIn.Read(1);
}
Solution 2
thanx for the help ppl, this was my first post and stackoverflow is awesome!
Also, I figured out another way to do this thing, using the oShell.SendKeys()
method.
Here's How:
var oShell = WScript.CreateObject("WScript.Shell");
oShell.SendKeys("cls{enter}timeout /t 10{enter}");
This way you can run almost every dos command without spawning a new process or window
EDIT: Although it seems to solve the problem, this code is not very reliable. See the comments below
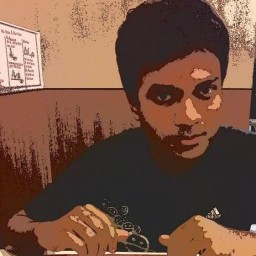
detj
!''# ^"`$$- !=@$_ %*~4 &[]../ |{,,SYSTEM HALTED How to read Waka waka bang splat tick tick hash, Caret quote back-tick dollar dollar dash, Bang splat equal at dollar under-score, Percent splat waka waka tilde number four, Ampersand bracket bracket dot dot slash, Vertical-bar curly-bracket comma comma CRASH
Updated on June 19, 2022Comments
-
detj 11 months
I'm writing a JScript program which is run in cscript.exe. Is it possible to run a commnad line command from within the script. It would really make the job easy, as I can run certain commands instead of writing more code in jscript to do the same thing.
For example: In order to wait for a keypress for 10 seconds, I could straight away use the timeout command
timeout /t 10
Implementing this in jscript means more work. btw, I'm on Vista and WSH v5.7
any ideas? thanx!
-
Helen over 13 yearsYour SendKeys string is incorrect - it should be "cls{enter}timeout /t 10{enter}" (without pluses).
-
Helen over 13 yearsAlso, your variant is very unreliable. First of all,
SendKeys
sends keystrokes to the active window, so the input might be sent to another window that has been accidentally activated. Also, if there're commands followingSendKeys
, they won't nesessary be executed afterSendKeys
. Try inserting theWScript.Echo
commands before and afterSendKeys
and removingcls
from the input string -- and you will see that the script output is messed up and thetimeout
command is executed last of all. -
detj over 13 yearsYeah..this code is really messy! I started experiencing problems once I ran it with the rest of the code and its true that with SendKeys, statements don't necessarily get executed as they appear. It gets even worse if you have a WScript.StdIn.Readline() with SendKeys(), as it wouldn't wait for the user to input coz it's already getting input from SendKeys and it does break up things the nasty way! Lesson learnt: Be very careful with SendKeys, it can be sloppy great insight helen!