Is there a way to save [[Promise Result]] as a variable?
16,929
Solution 1
There are 3 ways for solving this:
- Since you return a promise, use
.then
to get the returned value.
function currentloginid() {
return fetch('http://localhost/gaq/api/api.php?action=userid', {
method: 'GET',
})
.then(function(response) {
return response.json();
})
.then(function(data) {
var userid = JSON.parse(data);
console.log(userid);
return userid;
})
}
currentloginid().then(value => console.log(value));
- In one of the
.then
you already have, set an outside variable to the value. However this solution is not good since you could encounter situation wheremyValue
is not set.
let myValue;
function currentloginid() {
return fetch('http://localhost/gaq/api/api.php?action=userid', {
method: 'GET',
})
.then(function(response) {
return response.json();
})
.then(function(data) {
var userid = JSON.parse(data);
console.log(userid);
myValue = userid
return userid;
})
}
currentloginid();
console.log(myValue);
- Use the syntactic sugar
async await
to "await" for the value to return. I think that this approach is much more readable and easy to use (behind the scenes it's the same as option 1).
function currentloginid() {
return fetch('http://localhost/gaq/api/api.php?action=userid', {
method: 'GET',
})
.then(function(response) {
return response.json();
})
.then(function(data) {
var userid = JSON.parse(data);
console.log(userid);
return userid;
})
}
console.log(await currentloginid());
Solution 2
Instead of promise chains you could use async/await
and return the data(userid) directly.
const currentloginid = async () => {
const response = await fetch('http://localhost/gaq/api/api.php?action=userid')
const data = await response.json()
//console.log(JSON.parse(data))
return JSON.parse(data)
}
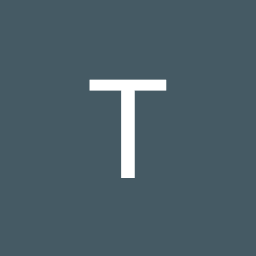
Author by
Tu-Ai Le
Updated on August 18, 2022Comments
-
Tu-Ai Le over 1 year
I am trying to access [[Promise Results]] and save it as a variable. The end goal I just want the result from the .then statement and use it in other function. If there is another better way to do it please let me, I'm new to JavaScript, so it would be awesome if you could explain it to me than just dump code. Thanks is advance Here is the fetch request
function currentloginid() { return fetch('http://localhost/gaq/api/api.php?action=userid', { method: 'GET', }) .then(function(response) { return response.json(); }) .then(function(data) { var userid = JSON.parse(data); console.log(userid); return userid; }) }
The code below is when I console log the function in another function
Promise {<pending>} __proto__: Promise [[PromiseState]]: "fulfilled" [[PromiseResult]]: 1
-
Tu-Ai Le over 3 yearsThank you!, you provided me with options of how to solve this problem which is great, and gave a brief explanation on how each of them worked, it help a lot thank you :)
-
EILYA over 2 yearsYou may need to use the "useState" and update the state in the chain.
-
charlietfl over 2 years@aminamin There is no React involved here
-
T. de Jong about 2 yearsThis explanation is great! I now grasp the concept of fetch way better!