Is there any easy way to change Spinner dropdown color in Android?
Solution 1
yes. You can use following attribute fro spinner inside your xml
android:popupBackground="YOUR_HEX_COLOR_CODE"
to change textcolor etc Make a custom XML file for your spinner item.
spin_item.xml:
Then provide it your desired color and sizes :
<?xml version="1.0" encoding="utf-8"?>
<TextView
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textSize="24sp"
android:textColor="#000000"
android:padding="4dp"
/>
And then use it like this:
val adapter = ArrayAdapter.createFromResource(activity,
R.array.email_type_array, android.R.layout.simple_spinner_item)
adapter.setDropDownViewResource(R.layout.spin_item)
Solution 2
To change the dropdown background color use android:popupBackground="@color/aColor"
on the xml file for your Spinner
widget:
<Spinner
android:id="@+id/my_spinner"
android:layout_width="100dp"
android:layout_height="match_parent"
android:popupBackground="@color/aColor" />
When playing with a light theme on your styles.xml
file the spinner dropdown icon color will be black, but pay attention that if you are using <item name="android:textColorSecondary">@color/aColor</item>
the dropdown icon will pick that color:
<!-- Base application theme. -->
<style name="AppTheme" parent="Theme.AppCompat.Light.NoActionBar">
<!-- Customize your theme here. -->
<item name="colorPrimary">@color/colorPrimary</item>
<item name="colorPrimaryDark">@color/colorPrimaryDark</item>
<item name="colorAccent">@color/colorAccent</item>
<item name="android:textColorSecondary">@color/aColor</item>
Even your question is about to change the dropdown background color I came here because I was trying to understand why my spinner dropdown icon color was with a different color until I discover that (android:textColorSecondary
) - So hope that helps someone else too.
Solution 3
Through code
Spinner spinner = (Spinner) findViewById(R.id.spinner);
spinner.getBackground().setColorFilter(getResources().getColor(R.color.red), PorterDuff.Mode.SRC_ATOP);
or Through XML
For API 21+:
<Spinner
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:backgroundTint="@color/red" />
or if you use the support library, you can use:
<android.support.v7.widget.AppCompatSpinner
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:backgroundTint="@color/red" />
Solution 4
In your code, add the following in your onCreate():
Spinner spinner = (Spinner) findViewById(R.id.spinner);
spinner.getBackground().setColorFilter(getResources().getColor(R.color.red),
PorterDuff.Mode.SRC_ATOP);
Solution 5
Create a new layout file which looks like this
<?xml version="1.0" encoding="utf-8"?>
<CheckedTextView xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@android:id/text1"
style="?android:attr/spinnerDropDownItemStyle"
android:singleLine="true"
android:layout_width="match_parent"
android:layout_height="?android:attr/dropdownListPreferredItemHeight"
android:ellipsize="marquee"
android:background="MY REQUIRED COLOR"/>
at place where i say MY REQUIRED COLOR
please set it to the color you want.
Also make sure that you dont change the android:id
attribute, because the arrayadapter is going to use this to set the text to the textview
Then set it to your arrayadapter during creation like so
val adapter = ArrayAdapter.createFromResource(activity,
R.array.email_type_array, .R.layout.custom_ simple_spinner_item)
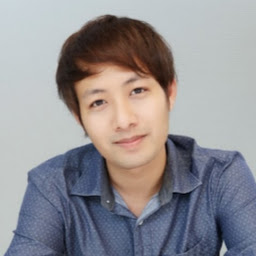
theerasan tonthongkam
Updated on June 12, 2022Comments
-
theerasan tonthongkam almost 2 years
I create my theme to use with the app and the parent of the theme is
Theme.AppCompat.Light.NoActionBar
by the way, I want white background and black text.
And this is adapter code
val adapter = ArrayAdapter.createFromResource(activity, R.array.email_type_array, android.R.layout.simple_spinner_item) adapter.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item) child.spinner.adapter = adapter
Is there any easy way to change Spinner dropdown color in Android?
-
theerasan tonthongkam over 6 yearsYeah, but the text still white
-
theerasan tonthongkam over 6 yearsNope, it changes the background of spinner itself, not the drop-down background color.
-
theerasan tonthongkam over 6 yearsNope, it changes the background of spinner itself, not the drop-down background color.
-
Akhilesh Awasthi over 6 yearsUpdated the answer. Please check.
-
theerasan tonthongkam over 6 yearsThank, Actually I've found another way, like this
android:popupBackground="YOUR_HEX_COLOR_CODE" <--- to set background android:popupTheme="@android:style/ThemeOverlay.Material.Dark" <--- to set text color
But I prefer to use your solution because of flexibility. I can set the style of text up to me, not have to follow Material theme