Is there any way to convert json to xml in PHP?
Solution 1
If you're willing to use the XML Serializer from PEAR, you can convert the JSON to a PHP object and then the PHP object to XML in two easy steps:
include("XML/Serializer.php");
function json_to_xml($json) {
$serializer = new XML_Serializer();
$obj = json_decode($json);
if ($serializer->serialize($obj)) {
return $serializer->getSerializedData();
}
else {
return null;
}
}
Solution 2
It depends on how exactly you want you XML to look like. I would try a combination of json_decode()
and the PEAR::XML_Serializer
(more info and examples on sitepoint.com).
require_once 'XML/Serializer.php';
$data = json_decode($json, true)
// An array of serializer options
$serializer_options = array (
'addDecl' => TRUE,
'encoding' => 'ISO-8859-1',
'indent' => ' ',
'rootName' => 'json',
'mode' => 'simplexml'
);
$Serializer = &new XML_Serializer($serializer_options);
$status = $Serializer->serialize($data);
if (PEAR::isError($status)) die($status->getMessage());
echo '<pre>';
echo htmlspecialchars($Serializer->getSerializedData());
echo '</pre>';
(Untested code - but you get the idea)
Solution 3
Crack open the JSON with json_decode
, and traverse it to generate whatever XML you want.
In case you're wondering, there is no canonical mapping between JSON and XML, so you have to write the XML-generation code yourself, based on the needs of your application.
Solution 4
I combined the two earlier suggestions into:
/**
* Convert JSON to XML
* @param string - json
* @return string - XML
*/
function json_to_xml($json)
{
include_once("XML/Serializer.php");
$options = array (
'addDecl' => TRUE,
'encoding' => 'UTF-8',
'indent' => ' ',
'rootName' => 'json',
'mode' => 'simplexml'
);
$serializer = new XML_Serializer($options);
$obj = json_decode($json);
if ($serializer->serialize($obj)) {
return $serializer->getSerializedData();
} else {
return null;
}
}
Solution 5
A native approch might be
function json_to_xml($obj){
$str = "";
if(is_null($obj))
return "<null/>";
elseif(is_array($obj)) {
//a list is a hash with 'simple' incremental keys
$is_list = array_keys($obj) == array_keys(array_values($obj));
if(!$is_list) {
$str.= "<hash>";
foreach($obj as $k=>$v)
$str.="<item key=\"$k\">".json_to_xml($v)."</item>".CRLF;
$str .= "</hash>";
} else {
$str.= "<list>";
foreach($obj as $v)
$str.="<item>".json_to_xml($v)."</item>".CRLF;
$str .= "</list>";
}
return $str;
} elseif(is_string($obj)) {
return htmlspecialchars($obj) != $obj ? "<![CDATA[$obj]]>" : $obj;
} elseif(is_scalar($obj))
return $obj;
else
throw new Exception("Unsupported type $obj");
}
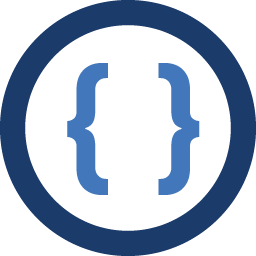
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
Is there any way to convert
json
toxml
inPHP
? I know that xml to json is very much possible. -
Ryan Maddox about 15 yearsAck, ya beat me by half a minute. I'll leave mine up anyway - it's a slightly different approach.
-
wired00 over 10 yearsPerfect for me Cheers