Is this the correct way to use an IllegalArgumentException?
Your TestScores
class should have two members: a constructor that accepts an array of scores and a method that returns the average of the scores. The assignment isn't totally clear as to which of these should throw an IllegalArgumentException
if a test score is out of range, but I'd make it the constructor (since that's what has the argument).
public class TestScores {
public TestScores(int[] scores) throws IllegalArgumentException {
// test the scores for validity and throw an exception if appropriate
// otherwise stash the scores in a field for later use
}
public float getAverageScore() {
// compute the average score and return it
}
}
You're on the right track with your TestScoresDemo
class. It will first need to collect a set of scores into an array. Then it should construct a TestScores
object. This is what needs to be inside a try
/catch
block because it can throw an exception. Then you just need to call getAverageScore()
and do something with the result.
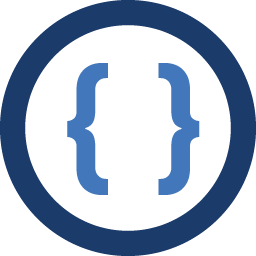
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I'm trying to work on a Java assignment. This is what it asks:
Write a class named
TestScores
. The class constructor should accept an array of the test scores as its argument. The class should have a method that returns the average of the test scores. If an test score in the array is negative or greater than 100, the class should throw anIllegalArgumentException
. Demonstrate. I need a file namedTestScores
andTestScoresDemo
.This is what I have so far. I know some of it is wrong and I need help fixing it:
class TestScores { public static void checkscore(int s) { if (s<0) throw new IllegalArgumentException("Error: score is negative."); else if (s>100) throw new IllegalArgumentException("Error Score is higher then 100"); else if (s>89)throw new IllegalArgumentException("Your grade is an A"); else if (s>79 && s<90)throw new IllegalArgumentException("Your grade is an B"); else if (s>69 && s<80)throw new IllegalArgumentException("Your grade is an C"); else if (s>59 && s<70)throw new IllegalArgumentException("Your grade is an D"); else if (s<60)throw new IllegalArgumentException("Your grade is an F"); { int sum = 0; //all elements together for (int i = 0; i < a.length; i++) sum += a[i]; } return sum / a.length; } } class TestScoresDemo { public static void main(String[] args) { int score = 0; Scanner scanner = new Scanner(System.in); System.out.print(" Enter a Grade number: "); String input = scanner.nextLine(); score = Integer.parseInt(input); TestScores.checkscore(score); System.out.print("Test score average is" + sum); } }
I know the assignment calls for a
try
statement because in my book that's what I see with theIllegalArgumentException
. Can anyone help me? I'm using Eclipse as an IDE.