Issue When Consume Rest Service with RestTemplate in Desktop App
13,783
Try using an array instead:
String url = "http://localhost:8080/mgm/country";
List<MediaType> mediaTypes = new ArrayList<MediaType>();
mediaTypes.add(MediaType.APPLICATION_JSON);
HttpHeaders headers = new HttpHeaders();
headers.setAccept(mediaTypes);
HttpEntity<Country> httpEntity = new HttpEntity<Country>(null, headers);
try {
ResponseEntity<Country[]> responseEntity = restTemplate.exchange(url, HttpMethod.GET, httpEntity, Country[].class);
Country[] countries = responseEntity.getBody();
System.out.println(countries[0].getName());
} catch (RestClientException exception) {
exception.printStackTrace();
}
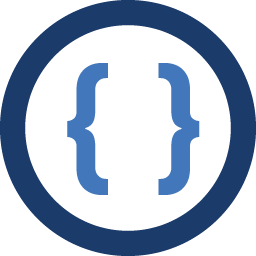
Author by
Admin
Updated on June 16, 2022Comments
-
Admin almost 2 years
I have a problem when Consume Rest Service with RestTemplate in Desktop App whereas the problem doesn't appear when i use in Web app.
This Is the Debugging logs
15:30:40.448 [main] DEBUG o.s.web.client.RestTemplate - Reading [java.util.List] as "application/json" using [org.springframework.http.converter.json.MappingJacksonHttpMessageConverter@98adae2] 15:30:40.452 [main] DEBUG httpclient.wire.content - << "[{"name":"Indonesia","id":1},{"name":"AlaySia","id":2},{"name":"Autraliya","id":3}]"
Exception in thread "main" java.lang.ClassCastException: java.util.LinkedHashMap cannot be cast to com.mgm.domain.Country
And this is the Code that i use.
String url = "http://localhost:8080/mgm/country"; List<MediaType> mediaTypes = new ArrayList<MediaType>(); mediaTypes.add(MediaType.APPLICATION_JSON); HttpHeaders headers = new HttpHeaders(); headers.setAccept(mediaTypes); HttpEntity<Country> httpEntity = new HttpEntity<Country>(null, headers); try { ResponseEntity<List> responseEntity = restTemplate.exchange(url, HttpMethod.GET, httpEntity, List.class); List<Country> countries = responseEntity.getBody(); System.out.println(countries.get(0).getName()); } catch (RestClientException exception) { exception.printStackTrace(); }
Code above doesn't give errors when i place it in web app. I use Spring Rest MVC to Provide JSON and Consume it with RestTemplate.
I think there is a problem when Jackson Convert
java.util.LinkedHashMap
toCountry
. it Seems thatcountries.get(0)
actually hasLinkedHashMap
type notCountry
and problem will appeared when i invoke one ofCountry
methode like.getName()
-
SingleShot over 12 years+1. Having the same issue, after some research on this I discovered your solution, came here to answer it, and see you beat me to it :-) The reason apparently is that you can't pass
List<Country>.class
to theexchange()
method, so when it unmarshalls it has no way to know the type (Country
) theList
is typed to. Arrays can be passed asClass
objects, so the type information can be conveyed to the underlying unmarshaller. -
Tobi Nonymous over 9 yearsyes, this is due to type erasure effects in the generics mechanism of Java. More found here: stackoverflow.com/questions/339699/… just adding my 2 cents