It is necessary to initialize Firebase Analytics in every Activity?
Solution 1
For screen reporting, you do not need to call FirebaseAnalytics.setCurrentScreen()
in every Activity because this is done for you automatically. The official docs state:
Note that screen reporting is enabled automatically and records the class name of the current Activity for you without requiring you to call this function.
Presumably, for this to work, you need to call FirebaseAnalytics.getInstance()
in your Application subclass onCreate()
method.
Solution 2
No. You just need to create global variable in an Class which extends Application class
public class MyApplication extends Application {
public static FirebaseAnalytics mFirebaseAnalytics;
@Override
public void onCreate() {
super.onCreate();
mFirebaseAnalytics = FirebaseAnalytics.getInstance(this);
}
}
After, you add the following line in your manifest, in the Application tag
<application
android:name=".MyApplication"
...
Solution 3
Screen tracking can now be done with only one line
**Your ApplicationClass**
public FirebaseAnalytics mFirebaseAnalytics;
@Override
public void onCreate() {
mFirebaseAnalytics = FirebaseAnalytics.getInstance(this);
}
public FirebaseAnalytics getmFirebaseAnalytics() {
return mFirebaseAnalytics;
}
After that create Base Activity and call the FirebaseAnalytics getter from here. Then use .setCurrentScreen as follows below
**Your BaseActivity**
@Override
protected void onResume() {
FirebaseAnalytics firebaseAnalytics = ((ApplicationClass) getApplication()).getmFirebaseAnalytics();
firebaseAnalytics.setCurrentScreen(this, getClass().getSimpleName(), null);
Log.d("FAnalytics", "setCurrentScreen: " + getClass().getSimpleName());
super.onResume();
}
Dont forget ! All your Activity has to be extends from BaseActivity https://firebase.google.com/docs/analytics/screenviews
Solution 4
Automatic Screen Tracking is not yet supported in Firebase Analytics, but this is something we are carefully considering right now.
Solution 5
Firebase Automatically tracks screens activities now, however, you can still track them manually.
mFirebaseAnalytics.setCurrentScreen(this, screenName, null /* class override */);
source:
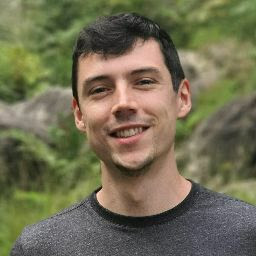
Óscar
As a software engineer specialized on Android Development, I work on developing applications that make life easier for people everyday.
Updated on June 03, 2022Comments
-
Óscar about 2 years
I don´t want to send any special logs to the Firebase Analytics console, just check in which screens is the user spending more time and so on.
When I used
AnalyticsTracker
it was compulsory to add it everywhere, so do you can set the specific name of every screen with theTracker.xml
file.The official documentation says:
Add the dependency for Firebase Analytics to your app-level
build.gradle
file:compile 'com.google.firebase:firebase-core:9.2.1'
Declare the FirebaseAnalytics object at the top of your activity:
private FirebaseAnalytics mFirebaseAnalytics;
Then initialize it in the
onCreate()
method:mFirebaseAnalytics = FirebaseAnalytics.getInstance(this);
So I guess I´ve to do this in every page where I want to get data, haven´t I?
-
Rony Tesler almost 8 yearsThen why did Steve Ganem say it's not supported?
-
RenatoIvancic almost 8 years@H.S.H is not saying that Page/ScreenViews are supported. He only answered the question if it needs to be initialized in every activity. And the answer is no, you can instantiate it in Application class.
-
Anuj over 6 yearsHello, i am Android noob, and am fascinated about what happened here. I think the real magic happened once
".MyApplication"
was added in manifest. Could you please take a moment and explain what exactly happened here? -
Anuj over 6 yearsAlso, i want to use the analytics instance to log different events, should i create a get method?
-
Tim over 5 yearsit is now, so you might want to delete this answer
-
tobltobs over 5 yearsThere is nothing mentioning a BaseActivity in the doc you are linking to. Could you explain what you mean with BaseActivity?
-
tobltobs over 5 years@Anuj
android:name=".MyApplication"
just tells Android that it should use theMyApplication
class as Application class. Without this your custom Application class would be ignored. And yes, if you want to log additional events you will have to create a getter. -
Emre Tekin over 5 yearsIf you use BaseActivity you need to call setCurrentScreen() just once. Otherwise you have to do that every activity. For Another solution using just once, you can open new class which is name AnalyticsHelper and put there method like public static void sendScreen(Activity activity, String eventName, String screenName) { ...} @tobltobs
-
Jaimin Modi almost 5 years@RonyTesler Because he thought that it's not supported.