Java 8 Stream API toMap converting to TreeMap
24,603
Solution 1
You can use overloaded groupingBy
method and pass TreeMap
as Supplier
:
TreeMap<User, List<Message>> map = list
.stream()
.collect(Collectors.groupingBy(Message::getSender,
() -> new TreeMap<>(new Usercomparator()), toList()));
Solution 2
If your list is sorted then just use this code for sorted map.
Map<String, List<WdHour>> pMonthlyDataMap = list
.stream().collect(Collectors.groupingBy(WdHour::getName, TreeMap::new, Collectors.toList()));
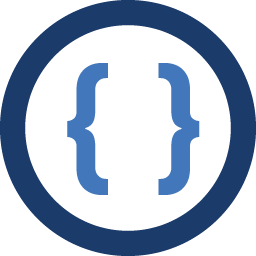
Author by
Admin
Updated on April 23, 2021Comments
-
Admin about 3 years
public class Message { private int id; private User sender; private User receiver; private String text; private Date senddate; .. }
I have
List<Message> list= new ArrayList<>();
I need to transform them to
TreeMap<User,List<Message>> map
I know how to do transform to HashMap using
list.stream().collect(Collectors.groupingBy(Message::getSender));
But I need TreeMap with: Key - User with newest message senddate first Value - List sorted by senddate newest first
Part of User class
public class User{ ... private List<Message> sendMessages; ... public List<Message> getSendMessages() { return sendMessages; } }
User comparator:
public class Usercomparator implements Comparator<User> { @Override public int compare(User o1, User o2) { return o2.getSendMessages().stream() .map(message -> message.getSenddate()) .max(Date::compareTo).get() .compareTo(o1.getSendMessages().stream() .map(message1 -> message1.getSenddate()) .max(Date::compareTo).get()); } }
-
Alexis C. about 8 yearsThe values won't be sorted.
-
Kashif Ibrahim over 4 yearsThis solution will give you the Map sorted by name, not by the sort order of the list.