Java Convert integer to hex integer
170,827
Solution 1
public static int convert(int n) {
return Integer.valueOf(String.valueOf(n), 16);
}
public static void main(String[] args) {
System.out.println(convert(20)); // 32
System.out.println(convert(54)); // 84
}
That is, treat the original number as if it was in hexadecimal, and then convert to decimal.
Solution 2
The easiest way is to use Integer.toHexString(int)
Solution 3
Another way to convert int to hex.
String hex = String.format("%X", int);
You can change capital X
to x
for lowercase.
Example:
String.format("%X", 31)
results 1F
.
String.format("%X", 32)
results 20
.
Solution 4
int orig = 20;
int res = Integer.parseInt(""+orig, 16);
Solution 5
You could try something like this (the way you would do it on paper):
public static int solve(int x){
int y=0;
int i=0;
while (x>0){
y+=(x%10)*Math.pow(16,i);
x/=10;
i++;
}
return y;
}
public static void main(String args[]){
System.out.println(solve(20));
System.out.println(solve(54));
}
For the examples you have given this would calculate: 0*16^0+2*16^1=32 and 4*16^0+5*16^1=84
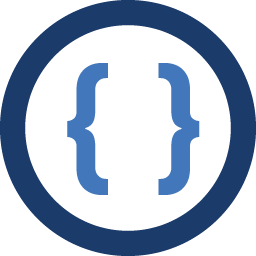
Author by
Admin
Updated on April 07, 2020Comments
-
Admin about 4 years
I'm trying to convert a number from an integer into an another integer which, if printed in hex, would look the same as the original integer.
For example:
Convert 20 to 32 (which is 0x20)
Convert 54 to 84 (which is 0x54)