Java/DOM: Get the XML content of a node
19,486
Solution 1
You have to use the transform/xslt API using your <b> node as the node to be transformed and put the result into a new StreamResult(new StringWriter()); . See how-to-pretty-print-xml-from-java
Solution 2
I know this was asked long ago but for the next person searching (was me today), this works with JDOM:
JDOMXPath xpath = new JDOMXPath("/td");
String innerXml = (new XMLOutputter()).outputString(xpath.selectNodes(document));
This passes a list of all child nodes into outputString, which will serialize them out in order.
Solution 3
What do you say about this ? I had same problem today on android, but i managed to make simple "serializator"
private String innerXml(Node node){
String s = "";
NodeList childs = node.getChildNodes();
for( int i = 0;i<childs.getLength();i++ ){
s+= serializeNode(childs.item(i));
}
return s;
}
private String serializeNode(Node node){
String s = "";
if( node.getNodeName().equals("#text") ) return node.getTextContent();
s+= "<" + node.getNodeName()+" ";
NamedNodeMap attributes = node.getAttributes();
if( attributes!= null ){
for( int i = 0;i<attributes.getLength();i++ ){
s+=attributes.item(i).getNodeName()+"=\""+attributes.item(i).getNodeValue()+"\"";
}
}
NodeList childs = node.getChildNodes();
if( childs == null || childs.getLength() == 0 ){
s+= "/>";
return s;
}
s+=">";
for( int i = 0;i<childs.getLength();i++ )
s+=serializeNode(childs.item(i));
s+= "</"+node.getNodeName()+">";
return s;
}
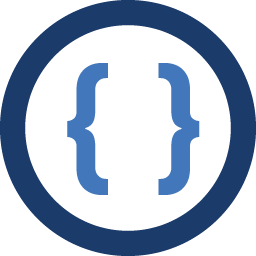
Author by
Admin
Updated on July 04, 2022Comments
-
Admin almost 2 years
I am parsing a XML file in Java using the W3C DOM. I am stuck at a specific problem, I can't figure out how to get the whole inner XML of a node.
The node looks like that:
<td><b>this</b> is a <b>test</b></td>
What function do I have to use to get that:
"<b>this</b> is a <b>test</b>"
-
Admin over 15 yearsNo? .toString() of my td-Node would just result in "[b: null]"
-
Jason S over 15 yearsHmm, I guess I got that confused with Javascript + e4x. I meant call the function which just produces the output, then delete the beginning/end tags.
-
Volodymyr Metlyakov over 5 yearsI say 'thanks a lot'! You saved my time from doing the same thing. Considering performance it is probably the most effective way.