Java for loop vs. while loop. Performance difference?
Solution 1
No, changing the type of loop wouldn't matter.
The only thing that can make it faster would be to have less nesting of loops, and looping over less values.
The only difference between a for
loop and a while
loop is the syntax for defining them. There is no performance difference at all.
int i = 0;
while (i < 20){
// do stuff
i++;
}
Is the same as:
for (int i = 0; i < 20; i++){
// do Stuff
}
(Actually the for-loop is a little better because the i
will be out of scope after the loop while the i
will stick around in the while
loop case.)
A for loop is just a syntactically prettier way of looping.
Solution 2
This kind of micro-optimization is pointless.
- A while-loop won’t be faster.
- The loop structure is not your bottleneck.
- Optimize your algorithm first.
- Better yet, don’t optimize first. Only optimize after you have found out that you really have a bottleneck in your algorithm that is not I/O-dependant.
Solution 3
Someone suggested to test while
vs for
loops, so I created some code to test whether while loops or for loops were faster; on average, over 100,000 tests, while
loop was faster ~95% of the time. I may have coded it incorrectly, I'm quite new to coding, also considering if I only ran 10,000 loops they ended up being quite even in run duration.
edit I didn't shift all the array values when I went to test for more trials. Fixed it so that it's easier to change how many trials you run.
import java.util.Arrays;
class WhilevsForLoops {
public static void main(String[] args) {
final int trials = 100; //change number of trials
final int trialsrun = trials - 1;
boolean[] fscount = new boolean[trials]; //faster / slower boolean
int p = 0; // while counter variable for for/while timers
while (p <= trialsrun) {
long[] forloop = new long[trials];
long[] whileloop = new long[trials];
long systimeaverage;
long systimenow = System.nanoTime();
long systimethen = System.nanoTime();
System.out.println("For loop time array : ");
for (int counter=0;counter <= trialsrun; counter++) {
systimenow = System.nanoTime();
System.out.print(" #" + counter + " @");
systimethen = System.nanoTime();
systimeaverage = (systimethen - systimenow);
System.out.print( systimeaverage + "ns |");
forloop[counter] = systimeaverage;
}
int count = 0;
System.out.println(" ");
System.out.println("While loop time array: ");
while (count <= trialsrun) {
systimenow = System.nanoTime();
System.out.print(" #" + count + " @");
systimethen = System.nanoTime();
systimeaverage = (systimethen - systimenow);
System.out.print( systimeaverage + "ns |");
whileloop[count] = systimeaverage;
count++;
}
System.out.println("===============================================");
int sum = 0;
for (int i = 0; i <= trialsrun; i++) {
sum += forloop[i];
}
System.out.println("for loop time average: " + (sum / trials) + "ns");
int sum1 = 0;
for (int i = 0; i <= trialsrun; i++) {
sum1 += whileloop[i];
}
System.out.println("while loop time average: " + (sum1 / trials) + "ns");
int longer = 0;
int shorter = 0;
int gap = 0;
sum = sum / trials;
sum1 = sum1 / trials;
if (sum1 > sum) {
longer = sum1;
shorter = sum;
}
else {
longer = sum;
shorter = sum1;
}
String longa;
if (sum1 > sum) {
longa = "~while loop~";
}
else {
longa = "~for loop~";
}
gap = longer - shorter;
System.out.println("The " + longa + " is the slower loop by: " + gap + "ns");
if (sum1 > sum) {
fscount[p] = true; }
else {
fscount[p] = false;
}
p++;
}
int forloopfc=0;
int whileloopfc=0;
System.out.println(Arrays.toString(fscount));
for(int k=0; k <= trialsrun; k++) {
if (fscount[k] == true) {
forloopfc++; }
else {
whileloopfc++;}
}
System.out.println("--------------------------------------------------");
System.out.println("The FOR loop was faster: " + forloopfc + " times.");
System.out.println("The WHILE loop was faster: " + whileloopfc + " times.");
}
}
Solution 4
you cant optimize it by changing it to while.
you can just increment speed very very very very little by changing the line
for (int k = 0; k < length - 1; k++) {
by
for (int k = 0; k < lengthMinusOne; k++) {
where lengthMinusOne is calculated before
this subtraction is just calculating almost (200x201/2) x (200-1) times and it is very little number for computer :)
Solution 5
here's a helpful link to an article on the matter
according to it, the While and For are almost twice as faster but both are the same.
BUT this article was written in 2009 and so i tried it on my machine and here are the results:
- using java 1.7: the Iterator was about 20%-30% faster than For and While (which were still the same)
- using java 1.6: the Iterator was about 5% faster than For and While (which were still the same)
so i guess the best thing is to just time it on your own version and machine and conclude from that
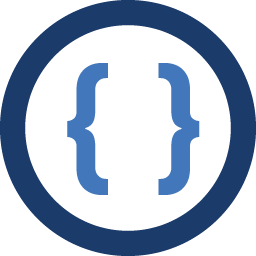
Admin
Updated on September 21, 2021Comments
-
Admin over 2 years
Assume i have the following code, there are three for loop to do something. Would it run fast if i change the most outer for loop to while loop? thanks~~
int length = 200; int test = 0; int[] input = new int[10]; for(int i = 1; i <= length; i++) { for (int j = 0; j <=length - i; j++) { for (int k = 0; k < length - 1; k++) { test = test + input[j + k]; } } }