Java - How Can I Write My ArrayList to a file, and Read (load) that file to the original ArrayList?
Solution 1
As an exercise, I would suggest doing the following:
public void save(String fileName) throws FileNotFoundException {
PrintWriter pw = new PrintWriter(new FileOutputStream(fileName));
for (Club club : clubs)
pw.println(club.getName());
pw.close();
}
This will write the name of each club on a new line in your file.
Soccer Chess Football Volleyball ...
I'll leave the loading to you. Hint: You wrote one line at a time, you can then read one line at a time.
Every class in Java extends the Object
class. As such you can override its methods. In this case, you should be interested by the toString()
method. In your Club
class, you can override it to print some message about the class in any format you'd like.
public String toString() {
return "Club:" + name;
}
You could then change the above code to:
public void save(String fileName) throws FileNotFoundException {
PrintWriter pw = new PrintWriter(new FileOutputStream(fileName));
for (Club club : clubs)
pw.println(club); // call toString() on club, like club.toString()
pw.close();
}
Solution 2
You should use Java's built in serialization mechanism. To use it, you need to do the following:
-
Declare the
Club
class as implementingSerializable
:public class Club implements Serializable { ... }
This tells the JVM that the class can be serialized to a stream. You don't have to implement any method, since this is a marker interface.
-
To write your list to a file do the following:
FileOutputStream fos = new FileOutputStream("t.tmp"); ObjectOutputStream oos = new ObjectOutputStream(fos); oos.writeObject(clubs); oos.close();
-
To read the list from a file, do the following:
FileInputStream fis = new FileInputStream("t.tmp"); ObjectInputStream ois = new ObjectInputStream(fis); List<Club> clubs = (List<Club>) ois.readObject(); ois.close();
Solution 3
In Java 8 you can use Files.write() method with two arguments: Path
and List<String>
, something like this:
List<String> clubNames = clubs.stream()
.map(Club::getName)
.collect(Collectors.toList())
try {
Files.write(Paths.get(fileName), clubNames);
} catch (IOException e) {
log.error("Unable to write out names", e);
}
Solution 4
This might work for you
public void save(String fileName) throws FileNotFoundException {
FileOutputStream fout= new FileOutputStream (fileName);
ObjectOutputStream oos = new ObjectOutputStream(fout);
oos.writeObject(clubs);
fout.close();
}
To read back you can have
public void read(String fileName) throws FileNotFoundException {
FileInputStream fin= new FileInputStream (fileName);
ObjectInputStream ois = new ObjectInputStream(fin);
clubs= (ArrayList<Clubs>)ois.readObject();
fin.close();
}
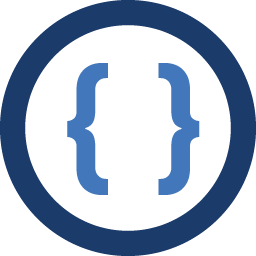
Admin
Updated on April 28, 2020Comments
-
Admin about 4 years
I am writing a program in Java which displays a range of afterschool clubs (E.G. Football, Hockey - entered by user). The clubs are added into the following
ArrayList
:private ArrayList<Club> clubs = new ArrayList<Club>();
By the followng Method:
public void addClub(String clubName) { Club club = findClub(clubName); if (club == null) clubs.add(new Club(clubName)); }
'Club' is a class with a constructor - name:
public class Club { private String name; public Club(String name) { this.name = name; } //There are more methods in my program but don't affect my query.. }
My program is working - it lets me add a new Club Object into my arraylist, i can view the arraylist, and i can delete any that i want etc.
However, I now want to save that arrayList (clubs) to a file, and then i want to be able to load the file up later and the same arraylist is there again.
I have two methods for this (see below), and have been trying to get it working but havent had anyluck, any help or advice would be appreciated.
Save Method (fileName is chosen by user)
public void save(String fileName) throws FileNotFoundException { String tmp = clubs.toString(); PrintWriter pw = new PrintWriter(new FileOutputStream(fileName)); pw.write(tmp); pw.close(); }
Load method (Current code wont run - File is a string but needs to be Club?
public void load(String fileName) throws FileNotFoundException { FileInputStream fileIn = new FileInputStream(fileName); Scanner scan = new Scanner(fileIn); String loadedClubs = scan.next(); clubs.add(loadedClubs); }
I am also using a GUI to run the application, and at the moment, i can click my Save button which then allows me to type a name and location and save it. The file appears and can be opened up in Notepad but displays as something like Club@c5d8jdj (for each Club in my list)