java: how do I create an array of tuples
Solution 1
Create a tuple class, something like:
class Tuple {
private Object[] data;
public Tuple (Object.. members) { this.data = members; }
public void get(int index) { return data[index]; }
public int getSize() { ... }
}
Then just create an array of Tuple instances.
Solution 2
if you want an arbitrary size tuple, perl hash style, use a Map<K,V>
(if you have a fixed type of keys values - your example looks like Map<Character,Integer>
would work - otherwise use the raw type). Look up the java collections for more details about the various implementations.
Given those tuples, if you want to stick them in an sequential collection, I'd use a List (again, look up the collections library).
So you end up with
List<Map<K,V>> listOfTuples
if you need something more specific (like, you'll always have x1, x2, x3 in your tuple) consider making the maps be EnumMaps - you can restrict what keys you have, and if you specify a default (or some other constraint during creation) guarantee that something will come out.
Solution 3
There's no default pair / n-tuple class in Java; you'd have to roll your own.
Solution 4
you could use the HashSet class.
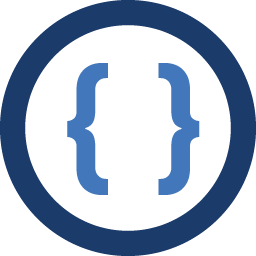
Admin
Updated on October 29, 2020Comments
-
Admin over 3 years
how can I create an array of tuples in jsp (java) like (a:1, b:2) (c:3, d:4) ... ...