Java. How to disable rounding in DecimalFormat
Solution 1
This is nothing to do with a feature of Java. It is due to the limited precision of IEEE 64-bit double floating numbers. In fact all data types have limits to their precision. SOme are larger than others.
double d = 123456789012345.99;
System.out.println(d);
prints
1.2345678901234598E14
If you want more precision use BigDecimal.
BigDecimal bd = new BigDecimal("123456789012345.99");
System.out.println(bd);
prints
123456789012345.99
Even BigDecimal has limits too, but they are far beyond what you or just about any one else would need. (~ 2 billion digits)
EDIT: The largest known primes fit into BigDecimal http://primes.utm.edu/largest.html
Solution 2
No, that's not rounding, that's just the way floating point numbers work.
The only way to do what you want is to use BigDecimal.
I'd recommend that you read "What Every Computer Scientist Should Know About Floating Point Arithmetic".
Solution 3
As far as I understood, you want to print the number in special format. You need to call applyPattern(String Pattern) as here:
f.applyPattern(".000");
System.out.println(f.format(123456789.99)); // prints 123456789.990
Note patterns are localised, e.g. in Germany it will print , instead of . to separate the fraction. You can set the rounding preferrence via
f.setRoundingMode()
Guess where did I learn all this? of course from Java docs. Read more about the pattern expression to format the way you like
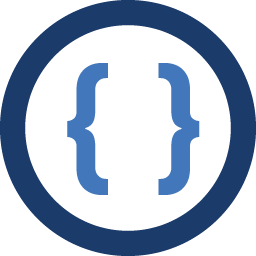
Admin
Updated on June 05, 2022Comments
-
Admin almost 2 years
Can I disable rounding "feature" in DecimalFormat?
For example:
DecimalFormat f = new DecimalFormat();
f.parseObject("123456789012345.99");
Result is 1.2345678901234598E14
java version "1.6.0_21"
-
jtahlborn about 13 yearsand, you can use DecimalFormat to parse a BigDecimal using
f.setParseBigDecimal(true)
. -
Vishy about 13 yearsWhat do you want to do that
new BigDecimal(String)
doesn't do? -
jtahlborn about 13 yearsi was just pointing out that DecimalFormat can parse into a BigDecimal as well. and, there are many formats that new BigDecimal can't handle (e.g. commas in the number).