Java how to replace 2 or more spaces with single space in string and delete leading and trailing spaces
Solution 1
Try this:
String after = before.trim().replaceAll(" +", " ");
See also
-
String.trim()
- Returns a copy of the string, with leading and trailing whitespace omitted.
- regular-expressions.info/Repetition
No trim()
regex
It's also possible to do this with just one replaceAll
, but this is much less readable than the trim()
solution. Nonetheless, it's provided here just to show what regex can do:
String[] tests = {
" x ", // [x]
" 1 2 3 ", // [1 2 3]
"", // []
" ", // []
};
for (String test : tests) {
System.out.format("[%s]%n",
test.replaceAll("^ +| +$|( )+", "$1")
);
}
There are 3 alternates:
-
^_+
: any sequence of spaces at the beginning of the string- Match and replace with
$1
, which captures the empty string
- Match and replace with
-
_+$
: any sequence of spaces at the end of the string- Match and replace with
$1
, which captures the empty string
- Match and replace with
-
(_)+
: any sequence of spaces that matches none of the above, meaning it's in the middle- Match and replace with
$1
, which captures a single space
- Match and replace with
See also
Solution 2
You just need a:
replaceAll("\\s{2,}", " ").trim();
where you match one or more spaces and replace them with a single space and then trim whitespaces at the beginning and end (you could actually invert by first trimming and then matching to make the regex quicker as someone pointed out).
To test this out quickly try:
System.out.println(new String(" hello there ").trim().replaceAll("\\s{2,}", " "));
and it will return:
"hello there"
Solution 3
Use the Apache commons StringUtils.normalizeSpace(String str)
method. See docs here
Solution 4
This worked perfectly for me : sValue = sValue.trim().replaceAll("\\s+", " ");
Solution 5
The following code will compact any whitespace between words and remove any at the string's beginning and end
String input = "\n\n\n a string with many spaces, \n"+
" a \t tab and a newline\n\n";
String output = input.trim().replaceAll("\\s+", " ");
System.out.println(output);
This will output a string with many spaces, a tab and a newline
Note that any non-printable characters including spaces, tabs and newlines will be compacted or removed
For more information see the respective documentation:
- String#trim() method
- String#replaceAll(String regex, String replacement) method
- For information about Java's regular expression implementation see the documentation of the Pattern class
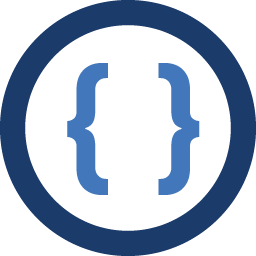
Admin
Updated on January 07, 2022Comments
-
Admin over 2 years
Looking for quick, simple way in Java to change this string
" hello there "
to something that looks like this
"hello there"
where I replace all those multiple spaces with a single space, except I also want the one or more spaces at the beginning of string to be gone.
Something like this gets me partly there
String mytext = " hello there "; mytext = mytext.replaceAll("( )+", " ");
but not quite.
-
corsiKa almost 14 years+1, especially as it's worth noting that doing
trim()
and thenreplaceAll()
uses less memory than doing it the other way around. Not by much, but if this gets called many many times, it might add up, especially if there's a lot of "trimmable whitespace". (Trim()
doesn't really get rid of the extra space - it just hides it by moving the start and end values. The underlyingchar[]
remains unchanged.) -
Marichyasana over 10 yearsI'm not an expert, but using your description of trim(), if the original string is deleted won't it also delete the trimmed string which is a part of it?
-
sp00m over 10 yearsIt's only a detail, but I think that
( ) +
or( ){2,}
should be a (very) little more efficient ;) -
djmj over 10 yearsNice regexp. Note: replacing the space ` ` with
\\s
will replace any group of whitespaces with the desired character. -
Lee Meador almost 8 yearsNote that the ( )+ part will match a single space and replace it with a single space. Perhaps (<space><space>+) would be better so it only matches if there are multiple spaces and the replacement will make a net change to the string.
-
Gary S. Weaver about 7 yearsAs Lee Meador mentioned,
.trim().replaceAll(" +", " ")
(with two spaces) is faster than.trim().replaceAll(" +", " ")
(with one space). I ran timing tests on strings that had only single spaces and all double spaces, and it came in substantially faster for both when doing a lot of operations (millions or more, depending on environment).