java.io.EOFException: End of input at line 1 column 1
When you see
java.io.EOFException: End of input at line 1 column 1
it indicates a problem with parsing something. It's expecting some text to parse but it got End of File (EOF).
Then you said:
When I changed server to external (nothing else changed)
If this worked before, your problem is definitely not on your code and it is on the data you're retrieving. Your new server is either rejecting your requests or returning blank data. Try doing the same request manually (via postman or some other api client) and see what the response is. It'll very likely tell you where the error is.
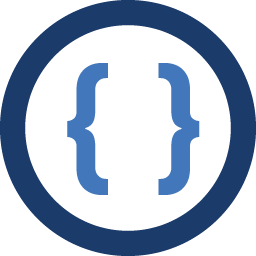
Admin
Updated on July 29, 2022Comments
-
Admin almost 2 years
My
Retrofit
should recieve a List of Bookmarks, and everything worked while I was usingWAMP
server. When I changed server to external (nothing else changed, just ip address of the server and retrieving of everything else works) I have an error:java.io.EOFException: End of input at line 1 column 1 at com.google.gson.stream.JsonReader.nextNonWhitespace(JsonReader.java:1407) at com.google.gson.stream.JsonReader.doPeek(JsonReader.java:553) at com.google.gson.stream.JsonReader.peek(JsonReader.java:429) at com.google.gson.internal.bind.CollectionTypeAdapterFactory$Adapter.read(CollectionTypeAdapterFactory.java:74) at com.google.gson.internal.bind.CollectionTypeAdapterFactory$Adapter.read(CollectionTypeAdapterFactory.java:61) at retrofit2.converter.gson.GsonResponseBodyConverter.convert(GsonResponseBodyConverter.java:37) at retrofit2.converter.gson.GsonResponseBodyConverter.convert(GsonResponseBodyConverter.java:25) at retrofit2.ServiceMethod.toResponse(ServiceMethod.java:116) at retrofit2.OkHttpCall.parseResponse(OkHttpCall.java:211) at retrofit2.OkHttpCall$1.onResponse(OkHttpCall.java:106) at okhttp3.RealCall$AsyncCall.execute(RealCall.java:135) at okhttp3.internal.NamedRunnable.run(NamedRunnable.java:32) at java.util.concurrent.ThreadPoolExecutor.runWorker(ThreadPoolExecutor.java:1112) at java.util.concurrent.ThreadPoolExecutor$Worker.run(ThreadPoolExecutor.java:587) at java.lang.Thread.run(Thread.java:818)
My Retrofit code:
public void init() { OkHttpClient.Builder okHttpClient = new OkHttpClient.Builder(); HttpLoggingInterceptor debugger = new HttpLoggingInterceptor() .setLevel(HttpLoggingInterceptor.Level.BODY); okHttpClient .addInterceptor(debugger); Retrofit retrofit = new Retrofit.Builder() .baseUrl(Constants.BASE_URL) .addConverterFactory(GsonConverterFactory.create()) .client(okHttpClient.build()) .build(); RequestInterface requestInterface = retrofit.create(RequestInterface.class); String email = pref.getString(Constants.EMAIL, ""); System.out.println(email); String id_group = pref.getString(Constants.ID_GROUP, ""); System.out.println(id_group); String nazwa = pref.getString(Constants.NAZWA, ""); Integer id_int_group = Integer.parseInt(id_group); Bookmark bookmark = new Bookmark(email, id_int_group, nazwa); ServerRequest request2 = new ServerRequest(); request2.setOperation(Constants.GET_MY_GROUPS); request2.setBookmark(bookmark); Call<List<Bookmark>> response2 = requestInterface.operation2(request2); response2.enqueue(new Callback<List<Bookmark>>() { @Override public void onResponse(Call<List<Bookmark>> call, retrofit2.Response<List<Bookmark>> response2) { listOfBookmarks = response2.body(); bookmarkToString(); simpleAdapter.notifyDataSetChanged(); // refresh listivew } @Override public void onFailure(Call<List<Bookmark>> call, Throwable t) { Log.d(Constants.TAG, "Nie zaladowano!", t); } }); }
EDIT:// PHP code:
<?php class Bookmark { private $host = 'localhost'; private $user = 'nwbrn_root'; private $db = 'nwbrn_app'; private $pass = 'zxs@1208NMLK'; private $conn; public function __construct() { $this -> conn = new PDO("mysql:host=".$this -> host.";dbname=".$this -> db, $this -> user, $this -> pass); } public function checkBookmarkExist($email, $id_group){ try { $query = $this->conn->prepare("SELECT COUNT(*) from bookmarks WHERE email =:email AND id_group =:id_group"); // $query = $this -> conn -> prepare($sql); $query->bindParam(':email', $email, PDO::PARAM_STR); $query->bindParam(':id_group', $id_group, PDO::PARAM_INT); $query->execute(array('email' => $email, 'id_group' => $id_group)); $row_count = $query -> fetchColumn(); if ( $row_count>0 ) { $response["result"] = "success"; $response["message"] = "Your favourite!"; return json_encode($response); } else { $response["result"] = "failure"; $response["message"] = "Not in your favourite!"; return json_encode($response); } } catch (PDOException $e) { die ($e->getMessage()); } } public function fullStarSelected($email, $id_group, $nazwa){ try { $query = $this->conn->prepare("DELETE from bookmarks WHERE email =:email AND id_group =:id_group AND nazwa =:nazwa"); // mysqli_set_charset($this->conn, "utf8"); $query->bindParam(':email', $email, PDO::PARAM_STR); $query->bindParam(':id_group', $id_group, PDO::PARAM_INT); $query->bindParam(':nazwa', $nazwa, PDO::PARAM_STR); $query->execute(); if ( $query ->rowCount() > 0 ) { $response["result"] = "failure"; $response["message"] = "Row not deleted!"; return json_encode($response); } else { $response["result"] = "success"; $response["message"] = "Row deleted successfully!"; return json_encode($response); } } catch (PDOException $e) { die ($e->getMessage()); } } public function blankStarSelected($email, $id_group, $nazwa){ try { $query = $this->conn->prepare("INSERT INTO bookmarks (email, id_group, nazwa) VALUES (:email, :id_group, :nazwa)"); // mysqli_set_charset($this->conn, "utf8"); $query->bindParam(':email', $email, PDO::PARAM_STR); $query->bindParam(':id_group', $id_group, PDO::PARAM_INT); $query->bindParam(':nazwa', $nazwa, PDO::PARAM_STR); $query->execute(); if (!$query) { printf("Error: %s\n", mysqli_error($this->conn)); exit(); } $result = array(); // $query1 = $this->conn->prepare("SELECT COUNT(*) from bookmarks WHERE email =:email AND id_group =:id_group LIMIT 1"); if ( $query->rowCount() > 0 ) { $response["result"] = "success"; $response["message"] = "Row added successfully!"; return json_encode($response); } else { $response["result"] = "failure"; $response["message"] = "Row not added!"; return json_encode($response); } } catch (PDOException $e) { die ($e->getMessage()); } } public function getMyGroups($email, $id_group){ try { $con = mysqli_connect($this->host,$this->user,$this->pass,$this->db); $sql = "SELECT * FROM bookmarks WHERE email = '$email'"; $res = mysqli_query($con,$sql); $result = array(); if (!$res) { printf("Error: %s\n", mysqli_error($con)); exit(); } while($row = mysqli_fetch_array($res)){ $temp = array(); $temp['id_group']=$row['id_group']; $temp['email']=$row['email']; $temp['nazwa']=$row['nazwa']; array_push($result,$temp); } echo json_encode($result); } catch (PDOException $e) { die ($e->getMessage()); } } }