Java JDBC: Reply.fill()
Solution 1
This is a sign of not properly closing/releasing JDBC resources. You need to acquire and close all JDBC resources in the shortest possible scope, i.e. you need to close them in the reversed order in the finally
block of the try
block of the very same method block as you've acquired them. E.g.
Connection connection = null;
Statement statement = null;
ResultSet resultSet = null;
try {
connection = database.getConnection();
statement = connection.createStatement();
resultSet = statement.executeQuery(SQL);
// ...
} finally {
if (resultSet != null) try { resultSet.close(); } catch (SQLException logOrIgnore) {}
if (statement != null) try { statement.close(); } catch (SQLException logOrIgnore) {}
if (connection != null) try { connection.close(); } catch (SQLException logOrIgnore) {}
}
If you don't close them properly as soon as possible, the DB will take it in own hands sooner or later and your application may break sooner or later as you encountered yourself.
To improve connecting performance, make use of a connection pool --you still need to acquire and close them in the same manner as here above though! It's now just the connection pool implementation which under the hoods worries about actually closing the connection or not.
Solution 2
we also recently faces this issue, and it was due to confusing AND OR clauses along with a and rownum < clause. Putting braces at the right places solved it.
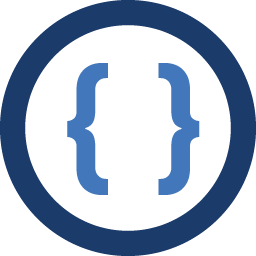
Admin
Updated on September 14, 2020Comments
-
Admin over 3 years
I get the following exception at times:
com.ibm.db2.jcc.b.gm: [jcc][t4][2030][11211][3.50.152] A communication error occurred during operations on the connection's underlying socket, socket input stream, or socket output stream. Error location: Reply.fill(). Message: Connection reset. ERRORCODE=-4499, SQLSTATE=08001
The problem is that, the code executes successfully for quite some time and then suddenly I get this exception. However it runs perfrectly when I run the code again.
Could some one please tell me what could be wrong and provide me some pointers to resolve this.