java.lang.NoClassDefFoundError: com.google.android.gms.common.internal.zzd
Solution 1
After some research and hours of testing I finally realized that android has something called the DEX 64K Methods Limit. In simple words there's a limit you can reach when adding external libraries to your project. When you reaches that limit you might need to use multidex. Personally I decided to reduce the amount of libraries imported as some of them weren't really used or necesary. For further understanding of multidex you should read this,
http://developer.android.com/tools/building/multidex.html
Solution 2
3 Simple Steps:
Step#1: Multidexing is a new feature and so requires a support library to be compatible with pre-lollipop devices. You need to add the following to your gradle file dependencies:
compile 'com.android.support:multidex:1.0.0'
Step#2: Also enable multidex output in your gradle file:
android {
compileSdkVersion 21
buildToolsVersion "21.1.0"
defaultConfig {
...
minSdkVersion 14
targetSdkVersion 21
...
// Enabling multidex support.
multiDexEnabled true
}
}
Step#3: And then add the multidex support application to your manifest:
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.android.multidex.myapplication">
<application
...
android:name="android.support.multidex.MultiDexApplication">
...
</application>
Note: If your app already uses(extends) the Application class, you can override the attachBaseContext() method and call MultiDex.install(this) to enable multidex. For more information, see the MultiDexApplication reference documentation.
@Override
protected void attachBaseContext(Context context) {
super.attachBaseContext(context);
MultiDex.install(this);
}
Here are the same instructions for reference: https://developer.android.com/tools/building/multidex.html
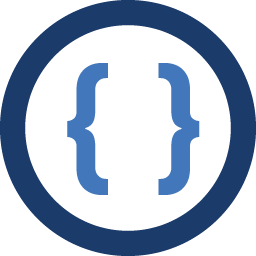
Admin
Updated on July 05, 2022Comments
-
Admin almost 2 years
Using Maps api v2 and it works perfectly with 4.4 , 5.0, 5.1, 5.1.1 , 6 but app crashes when I try 4.2.2. I've been doing lots of research but nothing seems to work. Here's the complete log.
FATAL EXCEPTION: main java.lang.NoClassDefFoundError: com.google.android.gms.common. at com.google.android.gms.measurement.internal.zzk$zza.get( at com.google.android.gms.measurement.internal.zzc.zzkG( at com.google.android.gms.measurement.internal.zzr.<init>( at com.google.android.gms.measurement.internal.zzx.zzb( at com.google.android.gms.measurement.internal.zzt.<init>( at com.google.android.gms.measurement.internal.zzx.zzBQ( at com.google.android.gms.measurement.internal.zzt.zzaU( at com.google.android.gms.measurement.ource) at android.content.ContentProvider.attachInfo(ContentProvider at android.app.ActivityThread.installProvider(ActivityThread. at android.app.ActivityThread.installContentProviders( at android.app.ActivityThread.handleBindApplication( at android.app.ActivityThread.access$1300(ActivityThread. at android.app.ActivityThread$H.handleMessage(ActivityThread. at android.os.Handler.dispatchMessage(Handler.java:99) at android.os.Looper.loop(Looper.java:137) at android.app.ActivityThread.main(ActivityThread.java:5041) at java.lang.reflect.Method.invokeNative(Native Method) at java.lang.reflect.Method.invoke(Method.java:511) at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run at com.android.internal.os.ZygoteInit.main(ZygoteInit. at dalvik.system.NativeStart.main(Native Method)
any ideas?
Here´s my build.gradle
buildscript { repositories { maven { url 'https://maven.fabric.io/public' } } dependencies { classpath 'io.fabric.tools:gradle:1.+' } } apply plugin: 'com.android.application' apply plugin: 'io.fabric' repositories { mavenCentral() maven { url "http://dl.bintray.com/glomadrian/maven" } maven { url 'https://maven.fabric.io/public' } } android { compileSdkVersion 23 buildToolsVersion "23.0.1" defaultConfig { applicationId "py.com.roshka.billeterabancard" minSdkVersion 14 targetSdkVersion 23 versionCode 1 versionName "1.0" multiDexEnabled true } buildTypes { release { minifyEnabled false proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.pro' } } } dependencies { compile fileTree(include: ['*.jar'], dir: 'libs') compile 'com.wang.avi:library:1.0.1' compile 'com.nineoldandroids:library:2.4.0' compile 'com.github.glomadrian:loadingballs:1.1@aar' compile 'com.android.support:appcompat-v7:23.1.0' compile 'com.google.android.gms:play-services:8.4.0' compile 'com.android.support:design:23.1.0' compile 'com.android.support:recyclerview-v7:23.1.0' compile 'com.squareup.okio:okio:1.0.+' compile 'com.squareup.okhttp:okhttp:1.5.4' compile 'com.squareup.okhttp:okhttp-urlconnection:2.0.0' compile 'com.squareup.retrofit:retrofit:1.8.0' compile 'com.pnikosis:materialish-progress:1.7' compile 'com.google.code.gson:gson:2.3' compile 'me.dm7.barcodescanner:zxing:1.8.3' compile 'me.dm7.barcodescanner:zbar:1.8.3' compile('com.crashlytics.sdk.android:crashlytics:2.5.5@aar') { transitive = true; } }