java.lang.NoSuchMethodError: Graph: method <init>()V not found
Solution 1
It looks like the eclipse has an old version of Graph.class
. You should try cleaning the project : See question Function of Project > Clean in Eclipse
Solution 2
Code compiles at command line but produces this error in Eclipse
Probable solutions : 1) Make sure the eclipse is using the same code as you use via command line. 2) Make a clean build inside eclipse because The case might be like it still referring the old class files regardless the thing that the source has been changed.
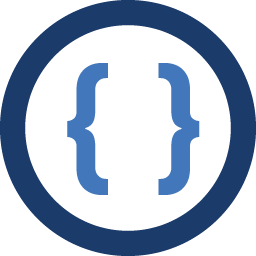
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I'm getting a confusing error in eclipse but not on our main server from the command line linux account. All code is in the main src directory, in eclipse. Code compiles at command line but produces this error in Eclipse on my Mac OS X laptop:
Exception in thread "main" java.lang.NoSuchMethodError: Graph: method <init>()V not found at Lab17.main(Lab17.java:11)
The code
Lab17.java
import java.io.IOException; import java.net.URL; import java.net.URLConnection; import java.util.Scanner; public class Lab17 { // Lab17 first attempt at creating a graph public static void main(String[] args) throws Exception { Graph myGraph = new Graph(); URLConnection conn = null; try { URL url = new URL("http://csc.mendocino.edu/~jbergamini/222data/flights/flights"); conn = url.openConnection(); } catch (IOException e) { System.out.println("Unable to open Flights file"); System.exit(1); } Scanner s = null; try { s = new Scanner(conn.getInputStream()); } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); } while (s.hasNext()) { String src = s.next(); String dst = s.next(); s.next(); double cost = s.nextDouble(); //System.out.println(src+" "+dst+" "+cost); myGraph.addEdge(src, dst, cost); } System.out.println(myGraph.breadthFirst("Austin", "Washington")); System.out.println(myGraph.depthFirst("LosAngeles", "Washington")); System.out.println(myGraph.breadthFirst("LosAngeles", "Washington")); System.out.println(myGraph.depthFirst("Washington", "LosAngeles")); System.out.println(myGraph.breadthFirst("Washington", "LosAngeles")); } }
Graph.java
import java.util.LinkedList; import java.util.Map; import java.util.NoSuchElementException; import java.util.TreeMap; public class Graph { private TreeMap<String, Vertex> vertexMap; /** Creates a new, empty graph */ public Graph() { // create vertexMap for Graph vertexMap = new TreeMap<String, Vertex>(); } /** * Adds an edge * @param src the source vertex * @param dst the destination vertex * @param cost the weight of the edge */ public void addEdge(String src, String dst, double cost) { //System.out.println(src+" "+dst+" "+cost+" in Graph()"); // check to see if there is a src vertex if(vertexMap.get(src) == null) { // if src Vertex does not exist create Vertex for src vertexMap.put(src, (new Vertex(src))); //System.out.println(vertexMap.get(src).toString()); } // check to see if there is a dst Vertex if(vertexMap.get(dst) == null) { // if dst Vertex does not exist create Vertex for dst vertexMap.put(dst, (new Vertex(dst))); //System.out.println(vertexMap.get(dst).toString()); } // populate the dst and cost for Edge on the src Vertex vertexMap element Vertex srdVertex = vertexMap.get(src); Vertex dstVertex = vertexMap.get(dst); Edge sEdge = new Edge(dstVertex, cost); srdVertex.addEdge(sEdge); } /** Clears/empties the graph */ public void clear() { vertexMap = new TreeMap<String,Vertex>(); } /** * Traverses, depth-first, from src to dst, and prints the vertex names in the order visited. * @param src the source vertex * @param dst the destination vertex * @return whether a path exists from src to dst */ public boolean depthFirst(String src, String dst) { System.out.println("Depth-first from "+src+" to "+dst); for(Map.Entry<String,Vertex> entry: vertexMap.entrySet()) { String key = entry.getKey(); Vertex thisVertex = entry.getValue(); System.out.println(key + " => " + thisVertex.toString()); } return false; } /** * Traverses, breadth-first, from src to dst, and prints the vertex names in the order visited * @param src the source vertex * @param dst the destination vertex * @return whether a path exists from src to dst */ public boolean breadthFirst(String src, String dst) { System.out.println("Breadth-first from "+src+" to "+dst); // find starting vertex in vertexMap Vertex start = vertexMap.get(src); LinkedList<Vertex> vertexQue = new LinkedList<Vertex>(); LinkedList<Vertex> visitedV = new LinkedList<Vertex>(); // check it for null if( start == null) { throw new NoSuchElementException(" Start vertex not found"); } // create a Queue for searching through vertex edges //Queue<Vertex> q = new Queue<Vertex>(); vertexQue.add( start ); start.dest = 0; boolean found = false; while( !vertexQue.isEmpty() && !found) { Vertex v = vertexQue.removeLast(); if( v.toString() == dst) { // print queue found = true; } else if(!visitedV.contains(v)){ // put all the adj vertex's into the queue for( Edge e: v.getEdges() ) { Vertex w = e.getDst(); vertexQue.add( w ); } } // add v to visitedV linked list if(!visitedV.contains(v)){ visitedV.add(v); } } System.out.print("["); for(int i=0; i < visitedV.size(); i++) { System.out.print(visitedV.get(i)+", "); } System.out.println("]"); /*forVertex> entry: vertexMap.entrySet()) { String key = entry.getKey(); Vertex thisVertex = entry.getValue(); System.out.println(key + " => " + thisVertex.toString()); for(Edge e : thisVertex.getEdges() ){ System.out.print(e.toString()); } System.out.println(); System.out.println("All Edges Evaluated"); }*/ return false; } }
Vertex.java
import java.util.Set; import java.util.TreeSet; public class Vertex { private String name; private TreeSet<Edge> adj; public double dest; /** * Creates a new vertex * @param name the name of the vertex */ public Vertex(String name) { this.name = name; adj = new TreeSet<Edge>(); } public TreeSet<Edge> getEdges() { return this.adj; } /** * Returns the set of all edges starting from this vertex. * Set shall be ordered with respect to the names of the destination vertices. */ public Set<Edge> allAdjacent() { Set<Edge> vertexSet = adj; return null; } public String toString() { return name; } public void addEdge(Edge e) { this.adj.add(e); } } public class Edge implements Comparable<Edge> { private Vertex dst; private double cost; /** Creates a new edge with an associated cost * @param dst the destination vertex * @param cost the weight of the edge */ public Edge(Vertex dst, double cost) { this.dst = dst; this.cost = cost; } public Vertex getDst() { return dst; } @Override public int compareTo(Edge o) { if(o == null) return -1; if(this == null) return -1; if( this.dst.toString().compareTo( ((Edge)o).dst.toString() ) == 0 ) return 0; else if ( this.dst.toString().compareTo( ((Edge)o).dst.toString() ) < 0 ) return 1; else return -1; } public String toString() { String theEdgeS = this.dst.toString()+" "+Double.toString(cost); return theEdgeS; } }
Not sure why Eclipse environment is treating the code differently than the command-line environment is. The class's are a work in progress for a class. I'm only trying to solve what seems like an Eclipse bug.