java nio and ByteBuffer problem
Solution 1
Whenever you read data into a buffer, if you want to write the buffer out another channel, you need to call: buffer.flip()
before writing. You don't need to duplicate the buffer if you are writing on the same thread as you are reading.
Also - BufferOverflowException means you are putting more data into the buffer than its capacity. This sounds like a buffer is not getting buffer.clear()
called to reset the position to zero.
There isn't enough code written above to diagnose exactly what the problem is.
Solution 2
Your program throws an exception while putting data so I would say that something is wrong with position/limit.
It looks like you are trying to put data into read buffer or trying to put more data than it's size. Buffer will not grow itself (ByteArrayOutputStream is better for this).
Read about clearing, rewinding and flipping in java documentation. This will reset position, limit or size of buffer.
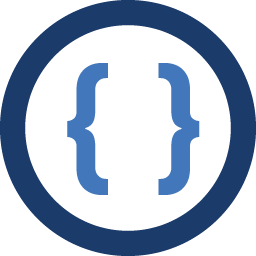
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I met a problem. I use nio socket to receive message. Upon a complete message is received, I send the dataBuffer which holds the received message to another user. But there is exception below. Where is the problem? I try to call dataBuffer.duplicate() and write it out. But at the receiver side, the read operation throws such exception. I have to assign a new ByteBuffer and make a new copy of message and write it out. In this case, there is no error. But I do not want the copy step. Is there any other way to solve it?
Exception thrown
java.nio.BufferOverflowException at java.nio.HeapByteBuffer.put(Unknown Source) at java.nio.ByteBuffer.put(Unknown Source) at serviceHandlerPackage.ServiceHandler.readComplete(ServiceHandler.java:218)
Code
readEventHAndler(SocketChannel socket) { readCompleteData(socket); } readCompleteData(Socket) { ByteBuffer dataBuffer; //hold complete message if(!dataComplete) return; else process(dataBuffer); } process(dataBuffer) { ... processHandler(); sendNext(dataBuffer); } sendNext(dataBuffer) { write(dataBuffer); }