Java secure session
Solution 1
You're still on the server while you invalidate the session.
//get stuff out of session you want before invalidating it.
currentSession = request.getSession(true);
UserProfile userProfile = (UserProfile) currentSession.getAttribute("userProfile");
//now invalidate it
currentSession.invalidate();
//get new session and stuff the data back in
HttpSession newSession = request.getSession(true);
newSession.setAttribute("userProfile", userProfile);
Solution 2
Get the existing; invalidate it; create a new one ...
1) Get the current Session with HttpServletRequest.getSession();
2) Clear the Session: HttpSession.invalidate();
3) Create a new one: HttpServletRequest.getSession(true);
Solution 3
Talking generally (because this isn't a Java problem at all, it's a general web problem) session fixation arises when session IDs are easy to discover or guess. The main method of attack is when the session ID is in the URL of a page, for example http://example.com/index?sessionId=123. An attacker could setup capture a session and then embed the link in their page, tricking a user into visiting it and becoming part of their session. Then when the user authenticates the session is authenticated. The mitigation for this is to not use URL based session IDs, but instead use cookies
Some web applications will use a cookie session based but set it from the initial URL, for example visiting http://example.com/index?sessionId=123 would see the session id in the url and then create a session cookie from it, setting the id in the session cookie to 123. The mitigation for this is to generate random session ids on the server without using any user input as a seed into the generator.
There's also browser based exploits where a poorly coded browser will accept cookie creation for domains which are not the originating domain, but there's not much you can do about that. And Cross Site Scripting attacks where you can send a script command into the attacked site to set the session cookie, which can be mitigated by setting the session cookie to be HTTP_ONLY (although Safari does not honour this flag)
For Java the general recommendation is
session.invalidate();
session=request.getSession(true);
However at one point on JBoss this didn't work - so you need to check this works as expected within your chosen framework.
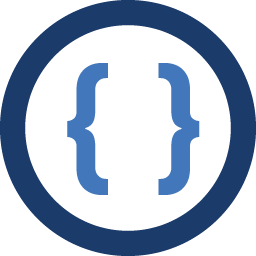
Admin
Updated on June 14, 2022Comments
-
Admin almost 2 years
Whenever you authenticate, your application should change the session identifier it uses. This helps to prevent someone from setting up a session, copying the session identifier, and then tricking a user into using the session. Because the attacker already knows the session identifier, they can use it to access the session after the user logs in, giving them full access. This attack has been called "session fixation" among other things. How can i change the session id once the user login to the system ?