Java: Splitting a string by whitespace when there is a variable number of whitespaces between words?
17,077
Solution 1
Use \\s+
to split on spaces even if they are more.
String string = "1-50 of 500+";
String[] stringArray = string.split("\\s+");
for (String str : stringArray)
{
System.out.println(str);
}
Full example: http://ideone.com/CFVr6N
EDIT:
If you also want to split on tabs, change the regex to \\s+|\\t+
and it detects both spaces and tabs as well.
Solution 2
You can split using regex
string.split("\\s+");
Solution 3
import java.util.*;
import java.lang.*;
import java.io.*;
/* Name of the class has to be "Main" only if the class is public. */
class Ideone
{
public static void main (String[] args) throws java.lang.Exception
{
String string = "1-50 of 500+";
String[] stringArray = string.split("\\s+");
for (String str : stringArray)
{
System.out.println(str);
}
}
}
Solution 4
Use this to spilt the String.
String[] stringArray = string.split("\\s+");
Solution 5
Your Program is correct but you put two space after 1-50
. So Its giving you that output. Remove one space and you are done. No need to change the code. But if there is a two space then you should use string.split("\\s+")
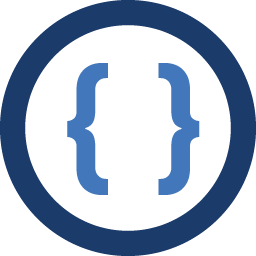
Author by
Admin
Updated on June 20, 2022Comments
-
Admin almost 2 years
I have the following:
String string = "1-50 of 500+"; String[] stringArray = string.split(" ");
Printing out all the elements in this array gives me the following:
Element 1: 1-50 Element 2: of 500+
How can I get it to split elements by the requirement that there is at least one whitespace between the words?
In other words, I want my elements to be:
Element 1: 1-50 Element 2: of Element 3: 500+
-
Admin over 10 yearsI might add I am retrieving the data from an HTML page... so maybe that space that I am seeing in the output is actually some other sort of whitespace?
-
Vimal Bera over 10 yearstry to use String#trim() function on every string from an array.
-
Pshemo about 10 yearsTab is considered whitespace so it belongs to
\s
class. There is no need to add special case for it.