Java System.currentTimeMillis() equivalent in C#
60,010
Solution 1
An alternative:
private static readonly DateTime Jan1st1970 = new DateTime
(1970, 1, 1, 0, 0, 0, DateTimeKind.Utc);
public static long CurrentTimeMillis()
{
return (long) (DateTime.UtcNow - Jan1st1970).TotalMilliseconds;
}
Solution 2
A common idiom in Java is to use the currentTimeMillis()
for timing or scheduling purposes, where you're not interested in the actual milliseconds since 1970, but instead calculate some relative value and compare later invocations of currentTimeMillis()
to that value.
If that's what you're looking for, the C# equivalent is Environment.TickCount
.
Solution 3
DateTimeOffset.UtcNow.ToUnixTimeMilliseconds()
Solution 4
If you are interested in TIMING, add a reference to System.Diagnostics and use a Stopwatch.
For example:
var sw = Stopwatch.StartNew();
...
var elapsedStage1 = sw.ElapsedMilliseconds;
...
var elapsedStage2 = sw.ElapsedMilliseconds;
...
sw.Stop();
Solution 5
We could also get a little fancy and do it as an extension method, so that it hangs off the DateTime class:
public static class DateTimeExtensions
{
private static DateTime Jan1st1970 = new DateTime(1970, 1, 1, 0, 0, 0, DateTimeKind.Utc);
public static long currentTimeMillis(this DateTime d)
{
return (long) ((DateTime.UtcNow - Jan1st1970).TotalMilliseconds);
}
}
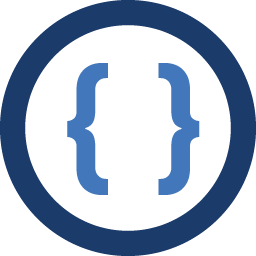
Author by
Admin
Updated on July 08, 2022Comments
-
Admin almost 2 years
What is the equivalent of Java's
System.currentTimeMillis()
in C#?