JAVA Variable declaration not allowed here
Solution 1
string
must be changed to String
.
By writing int score
you're trying to declare a new variable that already exists, which you declared before already. Just remove the int
part and you will get the assignment you want.
Solution 2
As per Java spec, You cannot declare a local variable when there is no scope. While declaring int score = 1
in if, there is no scope. http://docs.oracle.com/javase/specs/jls/se7/html/jls-6.html
A local variable, one of the following
*A local variable declared in a block
*A local variable declared in a for statement
Also you have already declared a variable named score
above. Even if you remove that declaration, you'll get the error because of the above reason.
Solution 3
Change int score = 1;
to score = 1;
.
Explanation:
To declare variable we use
someType variable;
To assign (or change) value to variable we use
variable = value;
We can mix these instruction into one line like;
someType variable = value;
So when you do
int score = 1;
you first declare variable score
and then assign 1
to it.
Problem here is that we can't have two (or more) local variables with same name in same scope. So something like
int x = 1;
int x = 2;
System.out.println(x)
is incorrect because we can't decide which x
we should use here.
Same about
int x = 1;
{
int x = 2;
System.out.println(x)
}
So if you simply want to change value of already created variable use only assignment, don't include declaration part (remove type information)
int x = 1;
//..
x = 2;//change value of x to 2
Now it is time for confusing part - scope. You need to understand that variable have some are in which they can be used. This area is called scope, and is marked with {
}
brackets which surrounds declaration of variable. So if you create variable like
{
int x = 1;
System.out.println(x); //we can use x here since we are in its scope
}
System.out.println(x); //we are outside of x scope, so we can't use it here
int x = 2;
System.out.println(x); //but now we have new x variable, so it is OK to use it
So because of that scope limitation declarations in places like
if (condition)
int variable = 2;
else
int variable = 3;
are incorrect because such code is equal to
if (condition){
int variable = 2;
}else{
int variable = 3;
}
so this variable couldn't be accessible anywhere.
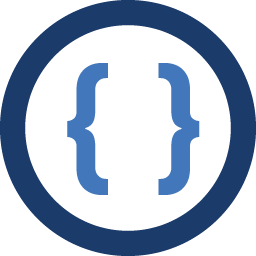
Admin
Updated on July 03, 2021Comments
-
Admin almost 3 years
I get an error "Variable declaration not allowed here" and I don't know why, I'm new in java and can't find answer :/ As it says, I can't make "int" in "if" but is there a way to create it?
import java.io.PrintWriter; import java.io.File; import java.io.FileNotFoundException; import java.util.Scanner;import java.util.Scanner; public class test{ public static void main(String[] args) throws FileNotFoundException{ File plik = new File("test.txt"); PrintWriter saver = new PrintWriter("test.txt"); int score = 0; System.out.println("Q: What's bigger"); System.out.println("A: Dog B: Ant"); Scanner odp = new Scanner(System.in); string odpo = odp.nextLine(); if(odpo.equals("a")) int score = 1; else System.out.println("Wrong answer"); } }