JavaFx: Button border and hover
42,025
Solution 1
Use the styles to remove the background :
.button {
-fx-background-color: transparent;
}
On hover, to bring back everything just use the button style from modena.css
:
.button:hover{
-fx-background-color: -fx-shadow-highlight-color, -fx-outer-border, -fx-inner-border, -fx-body-color;
-fx-background-insets: 0 0 -1 0, 0, 1, 2;
-fx-background-radius: 3px, 3px, 2px, 1px;
-fx-padding: 0.333333em 0.666667em 0.333333em 0.666667em; /* 4 8 4 8 */
-fx-text-fill: -fx-text-base-color;
-fx-alignment: CENTER;
-fx-content-display: LEFT;
}
Solution 2
I agree with netzwerg. If you are using fxml with javafx then you should include these lines of codes in initialize() method of your controller class.
Example of controller class:
public class Controller implements Initializable{
private Button test;
private static final String IDLE_BUTTON_STYLE = "-fx-background-color: transparent;";
private static final String HOVERED_BUTTON_STYLE = "-fx-background-color: -fx-shadow-highlight-color, -fx-outer-border, -fx-inner-border, -fx-body-color;";
@Override
public void initialize(URL location, ResourceBundle resources) {
button.setStyle(IDLE_BUTTON_STYLE);
button.setOnMouseEntered(e -> button.setStyle(HOVERED_BUTTON_STYLE));
button.setOnMouseExited(e -> button.setStyle(IDLE_BUTTON_STYLE));
}
}
Solution 3
Background transparency can be controlled through mouse listeners, i.e. by setting the CSS programmatically (which alleviates the need for an external stylesheet):
final String IDLE_BUTTON_STYLE = "-fx-background-color: transparent;";
final String HOVERED_BUTTON_STYLE = "-fx-background-color: -fx-shadow-highlight-color, -fx-outer-border, -fx-inner-border, -fx-body-color;";
button.setStyle(IDLE_BUTTON_STYLE);
button.setOnMouseEntered(e -> button.setStyle(HOVERED_BUTTON_STYLE));
button.setOnMouseExited(e -> button.setStyle(IDLE_BUTTON_STYLE));
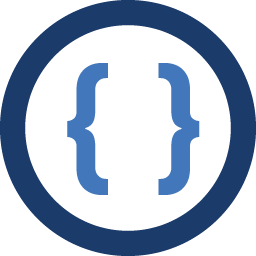
Author by
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I am using Java 8. I have toolbar and buttons on it.
I want to implement the following:
- In usual state (without mouse hover) at toolbar, only button label must be seen (no background, nor borders).
- When the user mouse hovers over the button, then the usual button must be seen.
How to do it via css?