Javascript - Array with Boolean Keys?
Solution 1
You can't use arbitrary indexes in an array, but you can use an object literal to (sort of) accomplish what you're after:
var test = {};
test[false] = "asdf";
test['false'] = "fdsa";
However it should be noted that object properties must be strings (or types that can be converted to strings). Using a boolean primitive will just end up in creating an object property named 'false'
.
test[false] === test['false'] === test.false
This is why your first example's Object.keys().length
call returns just 1
.
For an excellent getting started guide on objects in JavaScript, I would recommend MDN's Working with objects.
Solution 2
Arrays in Javascript aren't associative, so you cannot assign values to keys in them.
var test = [];
test.push(true); // [true]
test.push(false); // [true, false]
You're interested in an Object!
var test = {};
test[true] = "Success!";
test[false] = "Sadness"; // {'false': "Sadness", 'true': "Success"}
Solution 3
you could also reverse the answer above such as
let test = [true];
console.log(typeof test);
// Output: Object True
Solution 4
Javascript arrays are only number index based. You could use 0 and 1 as keys (although I can't think of a case where you need boolean keys). myArr[0] = "mapped from false"; myArr[1] = "mapped from true";
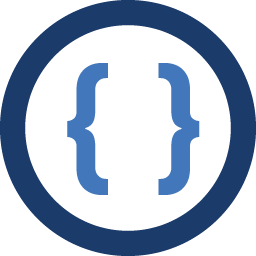
Admin
Updated on June 09, 2022Comments
-
Admin almost 2 years
I'm relatively new to Javascript, and there's probably just a trick I'm not familiar with, but how can I assign boolean values to Array keys?
What's happening:
var test = new Array(); test[false] = "asdf"; test['false'] = "fdsa"; Object.keys(test); // Yield [ "false" ] Object.keys(test).length; // Yield 1
What I want to happen:
var test = new Array(); //Some stuff Object.keys(test); // Yield [ "false" , false ] Object.keys(test).length; // Yield 2
-
Admin over 11 yearsThis is good to know. Maybe I'm just too spoiled for working mainly in PHP for the last year. I don't suppose I could have an array object with numeric and "associative" keys at the same time, could I? Basically, I need an array that holds single-level arrays, and if a user belongs to a certain group, a key is created in the upper-array to hold the lower-array of user information within that group, and if they're not a part of a group, they need to default to a "blank" group. The problem is that the group could be named anything, so I'd like this "blank" key to not be a string.
-
Andrew Whitaker over 11 yearsYou can name object properties integers (
var obj = { }; obj[1] = 'foo' }
). Typically you would use an object and then create a property of that object that's an array:var obj = { }; obj.ArrayProp = [];
. Hope that helps... -
Admin over 11 yearsThank you. People like you are the reason I love this site.