Javascript extracting number from string
Solution 1
First you need to convert the input prices from strings to numbers. Then subtract. And you'll have to convert the result back to "DKK ###,##" format. These two functions should help.
var priceAsFloat = function (price) {
return parseFloat(price.replace(/\./g, '').replace(/,/g,'.').replace(/[^\d\.]/g,''));
}
var formatPrice = function (price) {
return 'DKK ' + price.toString().replace(/\./g,',');
}
Then you can do this:
var productBeforePrice = "DKK 399,95";
var productCurrentPrice = "DKK 299,95";
productPriceDiff = formatPrice(priceAsFloat(productBeforePrice) - priceAsFloat(productCurrentPrice));
Solution 2
try:
var productCurrentPrice = productBeforePrice.replace(/[^\d.,]+/,'');
edit: this will get the price including numbers, commas, and periods. it does not verify that the number format is correct or that the numbers, periods, etc are contiguous. If you can be more precise in the exact number definitions you expcet, it would help.
Solution 3
try also:
var productCurrentPrice = productBeforePrice.match(/\d+(,\d+)?/)[0];
Solution 4
var productCurrentPrice = parseInt(productBeforePrice.replace(/[^\d\.]+/,''));
That should make productCurrentPrice the actual number you're after (if I understand your question correctly).
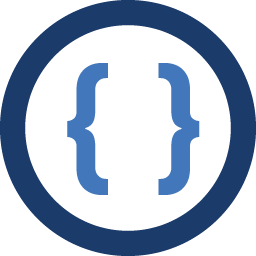
Admin
Updated on June 23, 2022Comments
-
Admin almost 2 years
I have a bunch of strings extracted from html using jQuery.
They look like this:
var productBeforePrice = "DKK 399,95"; var productCurrentPrice = "DKK 299,95";
I need to extract the number values in order to calculate the price difference.
(So I wend up with ≈
var productPriceDiff = DKK 100";
or just:
var productPriceDiff = 100";
)Can anyone help me do this?
Thanks, Jakob
-
brettkelly almost 15 yearsPretty sure that you need to escape that period, actually.
-
Pradeep Kumar almost 15 yearspretty sure you don't. it's inside the []. outside of that and it would need escaping. just tested in firefox and it's working as expected
-
Pradeep Kumar almost 15 yearsfails on commas as provided in his example of number format for danish kroners.
-
Admin almost 15 yearsWorks perfectly! (-: Thank you so much.
-
Admin almost 15 yearsJust realized it doesn't work with larger prices like "DKK 1.299,95".
-
Cédric Guillemette almost 15 yearsOkay, I fixed it to work with larger prices by stripping all of the periods before doing anything else. I didn't fix the formatPrice function. It will return a valid price, but without the periods ("DKK 1299,95"). If you need help doing that, ask another question. :-)