Javascript - get class owner of a function(method)
Solution 1
In javascript there are no Classes. Instead several objects can "own" the same function. For example:
function myFun(){
alert(this.name);
}
function Obj1(){
this.name = "obj1";
}
Obj1.prototype.fun = myFun;
function Obj2(){
this.name = "obj2";
}
Obj2.prototype.fun = myFun;
var obj1 = new Obj1();
var obj2 = new Obj2();
obj1.fun();
obj2.fun();
Solution 2
You scenario is not entirely clear but here are some options:-
function globalFunc()
{
alert(this.__class__);
//Note globalFunc now has access to much more.
}
function MyObject(){ }
MyObject.prototype.test=function(){
globalFunc.call(this);
}
MyObject.prototype.__class__=MyObject;
To add a closure for event handling
MyObject.prototype.test = function(){
var self = this;
var elem = //get some element;
//Not cross-browser but for illustration
elem.addEventListener('click', fnEvent);
function fnEvent() { globalFunc.call(self); }
elem = null
}
Solution 3
I don't understand well what you are trying to do, but here's an idea that could inspire you something.
The "constructor" property helped me well when I was trying to use JS as a OO language.
o.constructor
will give you the myObject
function reference.
But in my opinion, you should give functional programming a try instead of OO to get the most from Javascript
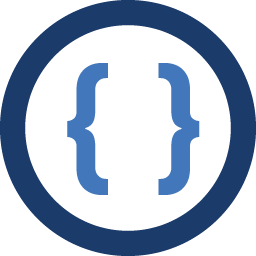
Admin
Updated on June 16, 2022Comments
-
Admin almost 2 years
there is a way to know what class own a function? Example:
function globalFunc(){ //alert MyObject } function MyObject(){ } MyObject.prototype.test=function(){ globalFunc(); } var o=new MyObject(); o.test(); //alert MyObject
Now im using this workaround:
function globalFunc(){ alert(globalFunc.caller.__class__); } function MyObject(){ } MyObject.prototype.test=function(){ globalFunc(); } MyObject.prototype.test.__class__=MyObject; var o=new MyObject(); o.test(); //alert MyObject
But there is a big problem, look this:
function globalFunc(){ alert(globalFunc.caller.__class__); } function MyObject(){ } MyObject.prototype.test=function(){ var temp=function(){ globalFunc(); } temp(); /* to simulate a simple closure, this may be for example: element.addEventListener("click",temp,false); */ } MyObject.prototype.test.__class__=MyObject; var o=new MyObject(); o.test(); //alert undefined
So, there is a clear way to obtain this? I know where is the problem(class property is a property of only test and not temp), but i can't add class to temp too.
Thanks.
Thanks for reply, some clarification.
Im trying to do a personal framwork OO oriented with private members.
So:
globalFunc is a special function, im using it to get "private" property and i can't call it with call method or passing some arguments, the only arguments im pass is "this":
Example, $() is global
Class({ public:{ MyClass:function(){ }, setName:function(name) { $(this).name=name; //set the private var name }, getName:function(){ return $(this).name; } }, private:{ name:"UNKNOWN" } }) var o=new MyClass(); o.getName(); // UNKNOWN o.setName("TEST!!!"); o.getName(); // TEST!!! o.name; //undefined $(o).name; //undefined
To works with inheritance, $(), i need to know what class call it and the object of the class.
All works good, but if i need to access a private members in a clousure i must add __class__ property to clouser!! And i not want this!
Thanks again and sorry for my bad english, im not native speaker.
-
Admin over 14 yearsYes..indeed im asking here if someone know another solution.
-
Admin over 14 yearsps. i cant add class property to temp too.
-
Marius about 9 years@CoDEmanX ES6 classes are just syntax sugar. Defining a method in a class is the same as setting the method on the prototype of the constructor