JavaScript input validation with regex
29,601
Firstly you need to cycle through each of your input elements, you can do this by using .each()
:
// Cycles through each input element
$(":input").each(function(){
var input = $(this).val();
...
});
Next your RegExp is only checking for the first character to be a letter, if you want to ensure that only a steam of letters can match you will want to use ^[a-zA-Z]+$
instead:
$(":input").each(function(){
var input = $(this).val();
var regex = new RegExp("^[a-zA-Z]+$");
if(regex.test(input)) {
alert("true");
}else {
alert("false");
return false;
}
});
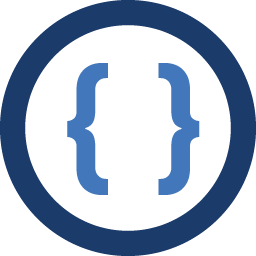
Author by
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I want to validate my inputs, so the user can just enter letters. The problem is that it just checks one input field but I selected all with
:input
code:
$('#formularID').submit(function() { var allInputs = $(":input").val(); var regex = new RegExp("[a-zA-Z]"); if(regex.test(allInputs)) { alert("true"); }else { alert("false"); return false; } });
I appreciate every help I can get!