Jenkins Pipeline passing password parameter to downstream job
Solution 1
You should make sure that parent and child job both are using password parameters. Then, this parameters tab will mask you password. Making build parameters as password parameter will not mask passwords in environment variables tab, for that you need to enable mask password in child and parent job configuration or use Inject passwords to the build as environment variables and enable mask password.
Solution 2
I've spent hours trying different solutions for the same problem as you've had and here is the final solution, which worked for me:
In your pipeline script:
stages {
stage("Do something with credentials and pass them to the downstream job") {
steps {
build job: 'your/jobname/path', parameters: [
[$class: 'hudson.model.PasswordParameterValue', name: 'PASSWORD', value: env.PASSWORD],
[$class: 'TextParameterValue', name: 'USERNAME', value: env.USERNAME]
]
}
}
The trick is to use hudson.model.PasswordParameterValue class when passing password parameter to downstream (Freestyle) job, but you must use then the same class for parameter definition in your main pipeline (parent job) in order to make it work.
For example in your pipeline job you would configure password parameter:
configure {
it / 'properties' / 'hudson.model.ParametersDefinitionProperty' / 'parameterDefinitions' << 'hudson.model.PasswordParameterDefinition' {
name('PASSWORD')
description('My password')
}
}
Solution 3
You should use credentials plugin, which in pipeline you write with withCredentials
block. For example:
withCredentials([usernamePassword(credentialsId: 'abcd1234-56ef-494f-a4d9-d5b5e8ac357d',
usernameVariable: 'USERNAME',
passwordVariable: 'PASSWORD')])
{
echo 'username='+USERNAME
echo 'password='+PASSWORD
}
where abcd1234-56ef-494f-a4d9-d5b5e8ac357d
is the id of credentials you have in jenkins, and of course, as long as you don't echo the variables (as I did in the example obviously for demonstration purposes), username and password are not visible.
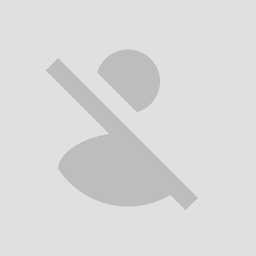
VladH
Updated on June 13, 2022Comments
-
VladH almost 2 years
I want to pass a value, from the Password Parameter plugin, in a Jenkins Pipeline job, to another freestyle job, to be used for login. I don't want to see it in the output or anywhere else. I can do it between two freestyle jobs but it seems that the pipeline is a bit different.
Even if I'm able to send as a string, it would be visible in the Parameters tab or the Environment Variables tab.
Does anyone have any idea how this could be achieved?
-
VladH over 6 yearsThank you for your suggestion, I tried that and it works. But I was wondering how I can achieve it without storying every single credential in Jenkins. Furthermore when I try to pass them down to my downstream job, these values are visible in the second job, Environment Variables tab.