JMeter : How to increment dates by +1 within date ranges for every iteration
Solution 1
For JMeter specifics:
-
Add User Defined Variables element to set initial date values like
- DATE1=2014/01/01
- DATE2=2014/09/01
-
Add a Beanshell PreProcessor as a child of the request where you need these updated dates. Put the following code into the PreProcessor's "Script" are:
import java.text.SimpleDateFormat; // necessary import java.util.Calendar; // imports import java.util.Date; SimpleDateFormat sdf = new SimpleDateFormat("yyyy/MM/dd"); // define date format Date date1 = sdf.parse(vars.get("DATE1")); // get DATE1 from User Defined variables Date date2 = sdf.parse(vars.get("DATE2")); // get DATE2 from UDV Calendar cal = Calendar.getInstance(); // get Calendar instance cal.setTime(date1); // set Calendar's time to be DATE1 cal.add(Calendar.DAY_OF_YEAR,1); // add 1 day to DATE1 date1 = cal.getTime(); // set the new value for date1 vars.put("DATE1",sdf.format(date1)); // update DATE1 JMeter variable cal.setTime(date2); // set Calendar time to DATE2 cal.add(Calendar.DAY_OF_YEAR,1); // add 1 day date2 = cal.getTime(); // set the new value for date2 vars.put("DATE2",sdf.format(date2)); // update DATE2 JMeter variable log.info("DATE1=" + vars.get("DATE1")); // print value of DATE1 to jmeter.log log.info("DATE2=" + vars.get("DATE2")); // print value of DATE2 to jmeter.log
Being executed for 3 times it provides the following output:
2014/08/30 10:11:13 INFO - jmeter.util.BeanShellTestElement: DATE1=2014/01/02
2014/08/30 10:11:13 INFO - jmeter.util.BeanShellTestElement: DATE2=2014/09/02
2014/08/30 10:11:13 INFO - jmeter.util.BeanShellTestElement: DATE1=2014/01/03
2014/08/30 10:11:13 INFO - jmeter.util.BeanShellTestElement: DATE2=2014/09/03
2014/08/30 10:11:13 INFO - jmeter.util.BeanShellTestElement: DATE1=2014/01/04
2014/08/30 10:11:13 INFO - jmeter.util.BeanShellTestElement: DATE2=2014/09/04
- Refer to the variables as
${DATE1}
and${DATE2}
where required.
For more information on Beanshell scripting in Apache JMeter check out How to use BeanShell: JMeter's favorite built-in component guide
Solution 2
In JMeter:
0/ First install Groovy:
Download it from http://groovy.codehaus.org/ , current version as of today is 2.3.6
Unzip the file and find folder embeddable and copy groovy-all-2.3.6.jar in jmeter/lib folder
Restart JMeter
That's it !
1/ Add User Defined Variables element to set initial date values like this:
- DATE1=2014/01/01
- DATE2=2014/09/01
2/ The recommended way to do this for REDUCED SYNTAX, PERFORMANCE and LANGUAGE POWER is to use Groovy + JSR223 PreProcessor, configure it this way:
3/ Paste this code in JSR 223 PreProcessor:
final String DATE_PATTERN = "yyyy/MM/dd";
def d1Plus1 = Date.parse(DATE_PATTERN, vars["DATE1"] ) + 1;
def d2Plus1 = Date.parse(DATE_PATTERN, vars["DATE2"] ) + 1;
vars.put("DATE1", d1Plus1.format(DATE_PATTERN));
vars.put("DATE2", d2Plus1.format(DATE_PATTERN));
4/ Refer to your variables as ${DATE1}
and ${DATE2}
5/ Running this Plan show this result:
For why Groovy is your best choice read this:
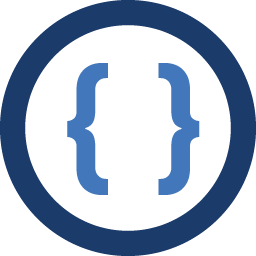
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I want to increment by 1 day 2 dates at every iteration using Jmeter i.e.
Date1 : 2014/01/01 (iteration1 = 2014/01/02) , (iteration1 = 2014/01/03) etc
Date 2 : 2014/09/01 (iteration1 = 2014/09/02) , (iteration1 = 2014/01/03) etc
How can I do it ?