Joining two ListViews into one in flutter
1,460
Solution 1
You can join two List
s into a single ListView
as follow:
Scaffold(
body: Column(
children: <Widget>[
Expanded(
child: ListView(
children: list1//list1 is a dummy list of String I created for demonstration
.map(
(listElement) => Text(//return your own widget instead of this
listElement,
style:
TextStyle(fontSize: 40, color: Colors.cyanAccent),
),
)
.toList()
..addAll(
list2.map(//list2 also is a dummy list of String I created for demonstration
(listElement) => Text(//return your own widget instead of this
listElement,
style: TextStyle(
fontSize: 40, color: Colors.cyanAccent),
),
),
),
),
),
],
),
),
Solution 2
Here is another way to make listview more flexible: DartPad
Use ...List
to connect multi-list:
Widget build(BuildContext context) {
// Demo listview items
List<String> list1 = [for (int i=0; i < 30;i++) i.toString()];
List<String> list2 = [for (int i=30; i < 50;i++) i.toString()];
return Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
body: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Expanded(
child: ListView(
children:[
// Some widgets here
const Text('List1'),
// list1 here
...list1.map((String text) => Text(text)).toList(),
// Some widgets between them
const Text('List2'),
// list2 here
...list2.map((String text) => Text(text)).toList(),
],),
),
],
),
);
}
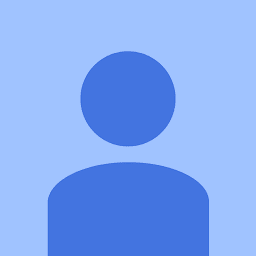
Author by
Ahmed Wagdi
Updated on December 19, 2022Comments
-
Ahmed Wagdi over 1 year
I have to list items in my flutter project and I need them to be viewed one after another using 2
ListView
, here is more details :lists
List<List<theLineModel.Line>> moreThenOneLineList = []; List<Station> mutualStationsList = []; List<theLineModel.Line> oneLineList = [];
The Widget
child: Column( children: <Widget>[ Expanded( child: ListView( children: oneLineList.map((line) { return GestureDetector( onTap: () { Navigator.pushNamed( context, '/line_details', arguments: { 'line': line, 'oneLine': true, }); }, child: Card( color: Colors.deepPurple, elevation: 8, child: Padding( padding: const EdgeInsets.all(8.0), child: Column( children: <Widget>[ Row( mainAxisAlignment: MainAxisAlignment .spaceEvenly, children: <Widget>[ Text( "${line .transportationType}", style: TextStyle( color: Colors.white, fontSize: 20), ), ], ), Row( mainAxisAlignment: MainAxisAlignment .center, children: <Widget>[ Text( "${line.startStation .stationName}", style: TextStyle( color: Colors.white, fontSize: 20), ), ], ), Row( mainAxisAlignment: MainAxisAlignment .center, children: <Widget>[ Icon( Icons.arrow_downward, color: Colors.white, size: 30, ) ], ), Row( mainAxisAlignment: MainAxisAlignment .center, children: <Widget>[ Text( "${line.endStation .stationName}", style: TextStyle( color: Colors.white, fontSize: 20), ), ], ), Row( mainAxisAlignment: MainAxisAlignment .spaceBetween, children: <Widget>[ Text( "${line.price} ${S .of(context) .le}", style: TextStyle( color: Colors.white, fontSize: 20), ), Text( "${line.notes}", style: TextStyle( color: Colors.white, fontSize: 20), ), ], ), ], ), ), ), ); }).toList(), ), ), Expanded( child: ListView( children: moreThenOneLineList .map((lineList) { return GestureDetector( onTap: () {}, child: Card( color: Colors.blueAccent, elevation: 8, child: Padding( padding: const EdgeInsets.all(8.0), child: Column( children: <Widget>[ Row( mainAxisAlignment: MainAxisAlignment .spaceEvenly, children: <Widget>[ Text( "${lineList[0] .transportationType}", style: TextStyle( color: Colors.white, fontSize: 20), ), ], ), Row( mainAxisAlignment: MainAxisAlignment .center, children: <Widget>[ Text( "${chosenStations[0] .stationName}", style: TextStyle( color: Colors.white, fontSize: 20), ), ], ), Row( mainAxisAlignment: MainAxisAlignment .center, children: <Widget>[ Icon( Icons.arrow_downward, color: Colors.white, size: 30, ) ], ), Row( mainAxisAlignment: MainAxisAlignment .center, children: <Widget>[ Text( "${mutualStationsList[moreThenOneLineList .indexOf( lineList)] .stationName}", style: TextStyle( color: Colors.white, fontSize: 20), ), ], ), Row( mainAxisAlignment: MainAxisAlignment .spaceBetween, children: <Widget>[ Text( "${lineList[0] .price} ${S .of(context) .le}", style: TextStyle( color: Colors.white, fontSize: 20), ), Text( "${lineList[0] .notes}", style: TextStyle( color: Colors.white, fontSize: 20), ), ], ), Icon( Icons.arrow_downward, color: Colors.white, size: 30, ), Row( mainAxisAlignment: MainAxisAlignment .spaceEvenly, children: <Widget>[ Text( "${lineList[1] .transportationType}", style: TextStyle( color: Colors.white, fontSize: 20), ), ], ), Row( mainAxisAlignment: MainAxisAlignment .center, children: <Widget>[ Text( "${mutualStationsList[moreThenOneLineList .indexOf( lineList)] .stationName}", style: TextStyle( color: Colors.white, fontSize: 20), ), ], ), Row( mainAxisAlignment: MainAxisAlignment .center, children: <Widget>[ Icon( Icons.arrow_downward, color: Colors.white, size: 30, ) ], ), Row( mainAxisAlignment: MainAxisAlignment .center, children: <Widget>[ Text( "${chosenStations[1] .stationName}", style: TextStyle( color: Colors.white, fontSize: 20), ), ], ), Row( mainAxisAlignment: MainAxisAlignment .spaceBetween, children: <Widget>[ Text( "${lineList[1] .price} ${S .of(context) .le}", style: TextStyle( color: Colors.white, fontSize: 20), ), Text( "${lineList[1] .notes}", style: TextStyle( color: Colors.white, fontSize: 20), ), ], ), ], ), ), ), ); }).toList(), ), ), ], ),
And then as both lists have been added to a different
ListView
so it caused space and bad looking page at the end, check the image.So, any idea how to add both lists to a single Listview?
-
user11073489 over 1 yearUsing the children property of ListView will build all the children at once