JPA string comparison
15,903
Solution 1
It might be a problem with string comparison in your JPA provider. Do you test it on the case-sensitive data?
You could also try (and it's the preferred way) using parameters instead of crafting your statement by hand. It's not only safer (prevents SQL injection) but also faster: not only for Java (you don't concatenate Strings) but also for the DB (the query can be prepared once for all executions). It might be something like this:
final String qstring = "SELECT e FROM Muser e WHERE e.name = :name";
TypedQuery<Muser> query = em.createQuery(qstring, Muser.class);
query.setParameter("name", name);
user = query.getSingleResult();
Solution 2
I think problem is because of String comparison.My solution to this problem is:
using lowercase for comparison.
final String qstring = "SELECT e FROM Muser e WHERE LOWER(e.name) = :name";
TypedQuery<Muser> query = em.createQuery(qstring, Muser.class);
query.setParameter("name", name.toLowerCase());
user = query.getSingleResult();
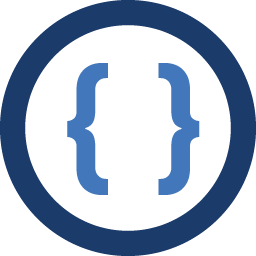
Author by
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I've written a simple login system using a JPQL query, which always returns no result:
public boolean check(String name, String password) { final String qstring="SELECT e FROM Muser e WHERE e.name = '"+name+"'"; Muser user; try{ user = em.createQuery(qstring, Muser.class).getSingleResult(); } catch(NoResultException e){ return false; } return password.equals(user.getPassword()); }
When I changed it to a native query:
user = (Muser) em.createNativeQuery(qstring, Muser.class).getSingleResult();
or an int expression:
final String qstring="SELECT e FROM Muser e WHERE e.id = "+id;
It goes all right. What's the problem? Thanks a million!