JPA with Hibernate 3 - ManyToMany-Stack overflow and Multiple bag errors
Solution 1
I've faced the similar issue and the root cause is a Lombok generation code as Vjeetje mentioned above. My code uses the annotation @Data, which generates hashCode and ToString methods with cross-dependent fields and this structure leads to Hibernate stuck. So, one should care to avoid these infinite loop calls, in my case I just added the exclusion parameters, like @EqualsAndHashCode(exclude="dependent_list") and @ToString(exclude = "dependent_list"). It solves stack overflow issue.
Solution 2
You cannot map the many to many relationship on both of the lists, hibernate will then try to fetch a collection for every nested element i.e. every user in the users list has a list of bank accounts which have a list of users... . think of it of a never ending recursion.
Solution 3
(Let's leave the fetch
attribute aside for now). Your mapping is perfectly valid and is the right way to map a bidirectional many-to-many relationship. From the JPA 2.0 specification:
2.10.4 Bidirectional ManyToMany Relationships
...
Example:
@Entity public class Project { private Collection<Employee> employees; @ManyToMany public Collection<Employee> getEmployees() { return employees; } public void setEmployees(Collection<Employee> employees) { this.employees = employees; } ... } @Entity public class Employee { private Collection<Project> projects; @ManyToMany(mappedBy="employees") public Collection<Project> getProjects() { return projects; } public void setProjects(Collection<Project> projects) { this.projects = projects; } ... }
In this example:
- Entity
Project
references a collection of EntityEmployee
.- Entity
Employee
references a collection of EntityProject
.- Entity
Project
is the owner of the relationship....
That being said, I'm unsure of the behavior when using EAGER
fetching on both sides (will it lead to an infinite cycle?), the JPA specification is pretty blurry about this and I can't find any clear mention that it is forbidden. But I bet that it's part of the problem.
But in the particular case of Hibernate, I'd expect Hibernate to be able to handle cycles as mentioned in this comment from Emmanuel Bernard:
LAZY or EAGER should be orthogonal to an infinite loop issue in the codebase. Hibernate knows how to handle cyclic graphs
Funnily enough, I've answered a very similar question recently (very close problem). Maybe an hint that something is wrong in Hibernate (my understanding of the above comment is that using EAGER fetching on both sides should work).
I'll thus conclude my answer in the same way: if you can provide a test case allowing to reproduce the problem, open a Jira issue.
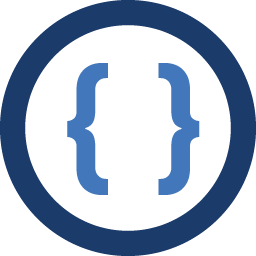
Admin
Updated on June 24, 2022Comments
-
Admin almost 2 years
I am facing issues while retrieving data for entities having bi-directional many-to-many relationship. If I use
List
for storing entities, I get unable to fetch multiple bags simultaneously error. If i change my code to useSet
, I get stackoverflow error.Details :
- Spring 3.0.3
- Hibernate-core : 3.5.1-Final
- Hibernate-annotations : 3.5.1-Final
- hibernate-common-annotations : 3.2.0-Final
- hibernate-entitymanager : 3.5.1-Final
- Mysql database
- Junit 4
User has Many Bank Accounts; Bank Account can have many users
User.java
@ManyToMany(fetch = FetchType.EAGER, mappedBy="user") private List<BankAccount> bankAccounts = new ArrayList<BankAccount>();
BankAccount.java
@ManyToMany(fetch = FetchType.EAGER) @JoinTable(name = "user_bankaccount", joinColumns = @JoinColumn(name="bank_account_id"), inverseJoinColumns = @JoinColumn(name = "user_id") ) private List<User> user = new ArrayList<User>();
DB Tables
Users user_id PK Bankaccount bank_account_id PK user_bankaccount bank_account_id PK ( references bankaccount.bank_account_id ) user_id PK ( references user.user_id )
issues
- when I try to get all the users data (
getAllUsers
) using a JUnit test case, I get unable to fetch multiple bags simultaneously error. - If I use
Set
andHashSet
instead of List andArrayList
respectively, I get stackoverflow error.
Please help me and let me know if code is wrong or its a known hibernate issue with specific version of libs that I am using.
-
Admin over 13 yearsHi Noam thanks for your reply. But dont we need Collection on both sides since its a many-to-many relationship ?
-
Noam Nevo over 13 yearsin order for hibernate to map the collections only one side is required. from the hibernate annotaion docs: "As seen previously, the other side don't have to (must not) describe the physical mapping: a simple mappedBy argument containing the owner side property name bind the two."
-
Admin over 13 yearsI have added JIRA for this issue alongwith complete source code opensource.atlassian.com/projects/hibernate/browse/HHH-5691
-
Admin over 13 yearsI was able to resolve the issue by removing references of other entity from toString() method. i.e removed references of BankAccount from User object's toString method and vice versa. I also removed fetch=fetchType.EAGER from all the entities.However, i thought that hibernate was able to detect such a cyclic dependency and not throw stackoverflow exception.
-
Vjeetje over 8 yearsI had a very similar issue using Lombok's ToSring annotation on a many-to-many relationship between two entities. Solution: use the exclude attribute of the ToString annotation. Anyway, Hibernate can work fine with a many-to-many relationship where both sides are fetched eagerly.
-
arnonuem about 4 yearsAn alternative is to annotate the fields with
@ToString.Exclude
and@EqualsAndHashCode.Exclude
-
kolya_metallist about 4 years@arnonuem Yes, but if Lombok version is 1.16.22 or higher