jquery 2.0 jquery ui datepicker bug - Uncaught TypeError: Cannot read property 'append' of undefined
Solution 1
One way that I've found to fix this problem, assuming you want to reset the datepicker is:
$(".hasDatepicker").removeClass("hasDatepicker");
$(".datepicker").datepicker("destroy");
$(".datepicker").datepicker();
Solution 2
This usually happens when you are calling destroy on a input which has the class, but does not have a datepicker() called on it yet. For example:
..input class="dp" />
If you haven't yet called $(".dp").datepicker()
and try to call $(".dp").datepicker("destroy")
you will get this error. This also happens when you are dynamically adding rows where one of the row fields is a datepicker input. In this case the code sequence should be:
$(".dp").datepicker("destroy");
// code to add row dynamically
.....
$(".dp").datepicker();
Solution 3
Bit late, but I removed the destroy function and got it working. Not exactly like your example, but this was gleamed from: JSFiddle
and removed the knoukout cleanup to get this solution:
ko.bindingHandlers.datepicker = {
init: function(element, valueAccessor, allBindingsAccessor) {
var $el = $(element);
//initialize datepicker with some optional options
var options = { minDate: 0
};
$el.datepicker(options);
//handle the field changing
ko.utils.registerEventHandler(element, "change", function() {
var observable = valueAccessor();
observable($el.datepicker("getDate"));
});
},
update: function(element, valueAccessor) {
var value = ko.utils.unwrapObservable(valueAccessor()),
$el = $(element),
current = $el.datepicker("getDate");
if (value - current !== 0) {
$el.datepicker("setDate", value);
}
}
};
I think the problem is that jquery shouldn't be forced to destroy the datepicker, when i removed this, i can refresh the page without the
Uncaught TypeError: Cannot read property 'append' of undefined
error
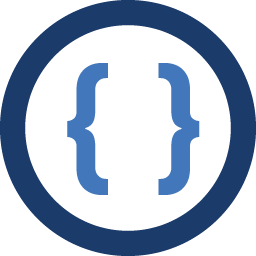
Admin
Updated on June 04, 2022Comments
-
Admin about 2 years
Since I've switched to JQuery 2.0 got a bug with destroying jQuery UI (1.10.2) Datepickers.
Seems like the problem in modification of jquery.each() function.
I do following
$(this.el_picker).datepicker('destroy');
it calls in JQuery UI line 9605
return this.each(function() { typeof options === "string" ? $.datepicker["_" + options + "Datepicker"]. apply($.datepicker, [this].concat(otherArgs)) : $.datepicker._attachDatepicker(this, options); });
Then it calls following in Jquery.each() function:
if ( isArray ) { for ( ; i < length; i++ ) { value = callback.call( obj[ i ], i, obj[ i ] ); if ( value === false ) { break; } } } else { for ( i in obj ) { value = callback.call( obj[ i ], i, obj[ i ] ); if ( value === false ) { break; } } }
And here is the difference with older version of JQuery
if ( isObj ) { for ( name in object ) { if ( callback.call( object[ name ], name, object[ name ] ) === false ) { break; } } } else { for ( ; i < length; ) { if ( callback.call( object[ i ], i, object[ i++ ] ) === false ) { break; } } } }
Then it goes to JQuery UI line 7922
_destroyDatepicker: function(target) { var nodeName, $target = $(target), inst = $.data(target, PROP_NAME); if (!$target.hasClass(this.markerClassName)) { return; } nodeName = target.nodeName.toLowerCase(); $.removeData(target, PROP_NAME); if (nodeName === "input") { inst.append.remove();
And throws error:
Uncaught TypeError: Cannot read property 'append' of undefined
Any ideas? Is it compatibility bug? How to fix it? Or I'm doing something wrong.