JQuery Blur Animation
10,438
Solution 1
Just change your function to this:
function blurElement(element, size) {
var filterVal = 'blur(' + size + 'px)';
$(element).css({
'filter':filterVal,
'webkitFilter':filterVal,
'mozFilter':filterVal,
'oFilter':filterVal,
'msFilter':filterVal,
'transition':'all 0.5s ease-out',
'-webkit-transition':'all 0.5s ease-out',
'-moz-transition':'all 0.5s ease-out',
'-o-transition':'all 0.5s ease-out'
});
}
Solution 2
In the css, give the #box
a transition:
#box{
filter: blur(0);
/* and the rest of it */
transition: 0.5s;
-webkit-transition:0.5s;
-moz-transition:0.5s;
}
Solution 3
Use javascript setTimeout:
setTimeout( function(){
$(element)
.css('filter',filterVal)
.css('webkitFilter',filterVal)
.css('mozFilter',filterVal)
.css('oFilter',filterVal)
.css('msFilter',filterVal);
}, 500);
If you were using jquery animations you could use .delay() but it won't work for css which isn't in the animation queue.
Solution 4
If you really want to use CSS3 use the below for either a box or text:
#box {
width:100px;
height:100px;
animation-name: blurbox;
animation-duration: 5s;
animation-fill-mode: forwards;
/* Chrome, Safari, Opera */
-webkit-animation-name: blurbox;
-webkit-animation-duration: 5s;
-webkit-animation-fill-mode: forwards;
/* Standard syntax */
-moz-animation-name: blurbox;
-moz-animation-duration: 5s;
-o-animation-name: blurbox;
-o-animation-duration: 5s;
-o-animation-fill-mode: forwards;
}
@-webkit-keyframes blurbox {
0% { background-color:#f00;}
100% { background-color:#f00; -webkit-filter: blur(50px);}
}
@-moz-keyframes blurbox {
0% { background-color:#f00;}
100% { background-color:#f00; -moz-filter: blur(50px); }
}
@keyframes blurbox {
0% { background-color:#f00; }
100% { background-color:#f00; filter: blur(50px); }
}
#text {
animation-name: blurtext;
animation-duration: 5s;
animation-fill-mode: forwards;
/* Chrome, Safari, Opera */
-webkit-animation-name: blurtext;
-webkit-animation-duration: 5s;
-webkit-animation-fill-mode: forwards;
/* Standard syntax */
-moz-animation-name: blurtext;
-moz-animation-duration: 5s;
-o-animation-name: blurtext; blurtext;
-o-animation-duration: 5s;
-o-animation-fill-mode: forwards;
}
@-webkit-keyframes blurtext {
0% { color: #000; }
100% { text-shadow: 0px 0px 10px #000; color: transparent; }
}
@-moz-keyframes blurtext {
0% { color: #000; }
100% { text-shadow: 0px 0px 10px #000; color: transparent; }
}
@keyframes blurtext {
0% { color: #000;}
100% { text-shadow: 0px 0px 10px #000; color: transparent; }
}
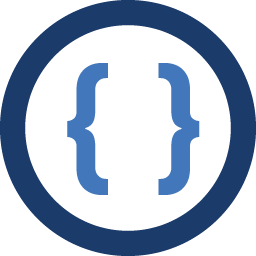
Author by
Admin
Updated on June 22, 2022Comments
-
Admin almost 2 years
I'm using the following script to blur a box when I click a button, but how can I make the blur take 500ms?
$(document).ready(function() { //attach click event to button $('.button').click(function(){ //when button is clicked we call blurElement function blurElement("#box", 2); }); //attach click event to button $('.button2').click(function(){ //when button is clicked we disable the blur blurElement("#box", 0); }); }); //set the css3 blur to an element function blurElement(element, size){ var filterVal = 'blur('+size+'px)'; $(element) .css('filter',filterVal) .css('webkitFilter',filterVal) .css('mozFilter',filterVal) .css('oFilter',filterVal) .css('msFilter',filterVal); } </script>
-
Pat Newell about 10 yearsI'm with you @BeatAlex... I highly recommend using jQuery simply to add a class to the element on which you want the transition to take place and let CSS do the work.
-
laaposto about 10 yearsThis will not create an animation. It will just blur the element 500ms later
-
Admin about 10 yearsI used this one, it was the easiest. Thanks
-
Stef Hej about 7 yearsWorks like a charme!