JQuery/Bootstrap: close and open the same modal to refresh the content, which is generated dynamically
Solution 1
Since you are hiding and showing on the same instance it will create one more backdrop but will not be able to show the modal
again. So you either can have a setTimeout
before showing the modal
with a minimal time interval, which will be inefficient as a backdrop will not be destroyed or just make use of shown.bs.modal
method and hide modal
before showing as below:
function openmodal(id){
var id=id;
//No need to hide here
$('#item_modal').modal('show');
$("#target_title").text(id);
}
$("#item_modal").on("shown.bs.modal",function(){
//will be executed everytime #item_modal is shown
$(this).hide().show(); //hide first and then show here
});
UPDATE
To have a fadeIn
and fadeOut
effect here is the little trick but you might feel its bit slow
and its upto you to opt this solution or not
function openmodal(id){
var id=id;
var other="<a onclick='openmodal(1)' style='cursor:pointer'>product 1</a><a onclick='openmodal(2)' style='cursor:pointer'>product 2</a><a onclick='openmodal(3)' style='cursor:pointer' >product 3</a>";
$('#item_modal').fadeOut("slow",function(){
$(this).modal('hide')
}).fadeIn("slow",function(){
$("#target_title").text(id);
$("#target_other").html(other);
$(this).modal('show')
});
}
You can now remove shown.bs.modal
part as it is handled in the function
itself
Solution 2
You can destroy the modal object before calling the same modal next time. The object is destroyed when the modal is hidden.
$('body').on('hidden.bs.modal', '.modal', function () {
$(this).removeData('bs.modal');
});
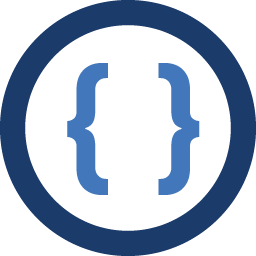
Admin
Updated on July 11, 2022Comments
-
Admin almost 2 years
I have a products page and once user click on a product I open up a modal generating dynamically the content. In the modal itself there are other products so when the user click there I would like to close the modal and re-open it in order to refresh the content - so once the modal reload the old content should be deleted and replaced with the new one.
Below a simplified version:
<html lang="en"> <head> <!-- Bootstrap Core CSS --> <link href="css/bootstrap.min.css" rel="stylesheet"> <script src="js/ajax.js"></script> <script> function openmodal(id){ var id=id; var other="<a onclick='openmodal(1)' style='cursor:pointer'>product 1</a><a onclick='openmodal(2)' style='cursor:pointer'>product 2</a><a onclick='openmodal(3)' style='cursor:pointer' >product 3</a>"; $('#item_modal').modal('show'); $("#target_title").text(id); $("#target_other").append(other); } </script> </head> <body> <a onclick="openmodal(1)" style="cursor:pointer">product 1</a> <a onclick="openmodal(2)" style="cursor:pointer">product 2</a> <a onclick="openmodal(3)" style="cursor:pointer">product 3</a> <div id="item_modal" class="modal fade" role="dialog"> <div class="modal-dialog"> <!-- Modal content--> <div class="modal-content"> <div class="modal-body"> <h1 id="target_title"></h1> <div id="target_other"> </div> </div> </div> </div> <!-- jQuery --> <script src="js/jquery.js"></script> <script src="js/bootstrap.min.js"></script> <script src="js/jquery.easing.min.js"></script> <script src="js/classie.js"></script> <script src="js/cbpAnimatedHeader.js"></script> </body> </html> </body> </html>
Any help? Thanks Best