jquery change image path
Solution 1
$('#bg img').attr('src', 'backgrounds/night.jpg')
should just be
$('#bg').prop('src', 'backgrounds/night.jpg')
As you specified the id
of the image already there is no need to include the img
. And .prop()
is preferred to attr()
as of jQuery 1.6
Solution 2
If it's :
<img src="someimage.jpg" id="bg" />
Then it's :
$('#bg').attr('src', 'backgrounds/night.jpg')
Solution 3
$('#bg').attr('src', function() {
var src = 'backgrounds/dawn.jpg';
if (hours > 20 || hours < 5) {
src = 'backgrounds/night.jpg';
} else if (hours > 17) {
src = 'backgrounds/dusk.jpg';
} else if (hours > 8) {
src = 'backgrounds/day.jpg';
}
return src;
})
Solution 4
You should probably use
$('#bg')
instead of
$('#bg img')
in the second case, you are selecting all the IMG elements wherever UNDER the element #bg.
Solution 5
Here is another more functional solution for your problem:
$("#bg").attr("src", function(i, value) {
var src = "backgrounds/";
if (hours > 20 || hours < 5) src += "night.jpg";
else if (hours > 17) src += "dusk.jpg";
else if (hours > 8) src += "day.jpg";
else src += "dawn.jpg";
return src;
});
And yes, you have to use #bg
selector, since this is image itself.
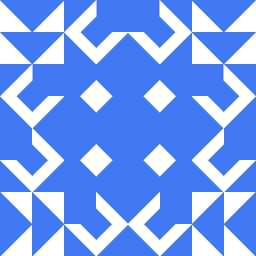
ecs
Updated on November 22, 2022Comments
-
ecs 6 months
I've had to use a div with an image to cover the page due to issues with getting the background to stretch across the entire page in different browsers.
What I'm trying to do is to get this image to change dependent on the time of day. I had it working fine when I was using the more conventional background-image property in CSS, but I'm having problems translating it over to change the src attribute of the image.
The id of the image is #bg. I'm probably making a syntactical error, but any help would be great, thanks!
$('document').ready(function(){ var hours = getDayTime(new Date().getHours()); if (hours > 20 || hours < 5) { $('#page-background > img').prop('src', 'backgrounds/night.jpg') } else if (hours > 17) { $('#page-background > img').prop('src', 'backgrounds/dusk.jpg') } else if (hours > 8) { $('#page-background > img').prop('src', 'backgrounds/day.jpg') } else { $('#page-background > img').prop('src', 'backgrounds/dawn.jpg') } }); <div id="page-background"><img src="backgrounds/day.jpg" width="100%" height="100%" alt="" id="bg" /></div>
-
hkutluay almost 11 yearswhere hours variable defined? can you put your whole page?
-
ecs almost 11 yearsI've added the relevant bits of HTML now as well, thanks.
-
Manse almost 11 years@EmilyTwist where is
hours
defined ? any errors ? -
ecs almost 11 years@ManseUK I've got it as:
code
$('document').ready(function(){ var hours = getDayTime(new Date().getHours()); if (hours > 20 || hours < 5) { $('#page-background > img').prop('src', 'backgrounds/night.jpg') }code
is that right?
-
-
ecs almost 11 yearsI've added the relevant bits of HTML and changed the code I'm trying - it's not working though - the image isn't changing in the browser :S
-
ecs almost 11 yearsI've changed how I'm doing it now, and edited my code with the changes people have suggested, but it still isn't working - any ideas?