jQuery count number of divs with a certain class?
Solution 1
You can use the jquery .length property
var numItems = $('.item').length;
Solution 2
For better performance you should use:
var numItems = $('div.item').length;
Since it will only look for the div
elements in DOM
and will be quick.
Suggestion: using size()
instead of length
property means one extra step in the processing since SIZE()
uses length
property in the function definition and returns the result.
Solution 3
You can use jQuery.children property.
var numItems = $('.wrapper').children('div').length;
for more information refer http://api.jquery.com/
Solution 4
And for the plain js answer if anyone might be interested;
var count = document.getElementsByClassName("item");
Cheers.
Reference: https://www.w3schools.com/jsref/met_document_getelementsbyclassname.asp
Solution 5
I just created this js function using the jQuery size function http://api.jquery.com/size/
function classCount(name){
alert($('.'+name).size())
}
It alerts out the number of times the class name occurs in the document.
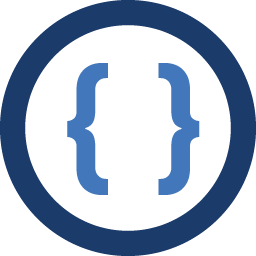
Admin
Updated on July 08, 2022Comments
-
Admin almost 2 years
Considering something like this;
<div class="wrapper"> <div class="item"></div> <div class="item"></div> <div class="item"></div> <div class="item"></div> <div class="item"></div> </div>
How would I, using jQuery (or plain JS, if it's shorter - but I doubt it) count the number of divs with the "item" class? In this example, the function should return 5, as there are 5 divs with the item class.
Thanks!