jQuery: get the file name selected from <input type="file" />
Solution 1
It is just such simple as writing:
$('input[type=file]').val()
Anyway, I suggest using name or ID attribute to select your input. And with event, it should look like this:
$('input[type=file]').change(function(e){
$in=$(this);
$in.next().html($in.val());
});
Solution 2
The simplest way is to simply use the following line of jquery
, using this you don't get the /fakepath
nonsense, you straight up get the file that was uploaded:
$('input[type=file]')[0].files[0]; // This gets the file
$('#idOfFileUpload')[0].files[0]; // This gets the file with the specified id
Some other useful commands are:
To get the name of the file:
$('input[type=file]')[0].files[0].name; // This gets the file name
To get the type of the file:
If I were to upload a PNG, it would return image/png
$("#imgUpload")[0].files[0].type
To get the size (in bytes) of the file:
$("#imgUpload")[0].files[0].size
Also you don't have to use these commands on('change'
, you can get the values at any time, for instance you may have a file upload and when the user clicks upload
, you simply use the commands I listed.
Solution 3
$('input[type=file]').change(function(e){
$(this).parents('.parent-selector').find('.element-to-paste-filename').text(e.target.files[0].name);
});
This code will not show C:\fakepath\
before file name in Google Chrome in case of using .val()
.
Solution 4
<input onchange="readURL(this);" type="file" name="userfile" />
<img src="" id="blah"/>
<script>
function readURL(input) {
if (input.files && input.files[0]) {
var reader = new FileReader();
reader.onload = function (e) {
$('#blah')
.attr('src', e.target.result)
.width(150).height(200);
};
reader.readAsDataURL(input.files[0]);
//console.log(reader);
//alert(reader.readAsDataURL(input.files[0]));
}
}
</script>
Solution 5
I had used following which worked correctly.
$('#fileAttached').attr('value', $('#attachment').val())
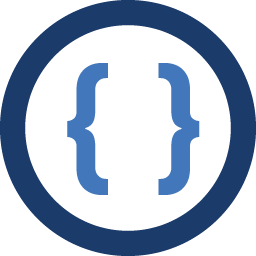
Admin
Updated on July 08, 2022Comments
-
Admin almost 2 years
This code should work in IE (don't even test it in Firefox), but it doesn't. What I want is to display the name of the attached file. Any help?
<html> <head> <title>example</title> <script type="text/javascript" src="../js/jquery.js"></script> <script type="text/javascript"> $(document).ready( function(){ $("#attach").after("<input id='fakeAttach' type='button' value='attach a file' />"); $("#fakeAttach").click(function() { $("#attach").click(); $("#maxSize").after("<div id='temporary'><span id='attachedFile'></span><input id='remove' type='button' value='remove' /></div>"); $('#attach').change(function(){ $("#fakeAttach").attr("disabled","disabled"); $("#attachedFile").html($(this).val()); }); $("#remove").click(function(e){ e.preventDefault(); $("#attach").replaceWith($("#attach").clone()); $("#fakeAttach").attr("disabled",""); $("#temporary").remove(); }); }) }); </script> </head> <body> <input id="attach" type="file" /><span id="maxSize">(less than 1MB)</span> </body> </html>
-
Admin almost 15 yearsThank you! That worked on the previous (and simplified) problem I posed, but it doen't work on the extended version.
-
Khurram Ijaz about 13 yearshow to get the complete path of the file the above code only gives the file name.
-
zneak over 12 years@PHP Priest, you can't. Browsers don't expose that kind of information.
-
jinglesthula over 12 years@PHP Priest, if you could do that, you could surreptitiously transmit data about the user's file system via AJAX and the user would likely never know. Imagine how much worse it would be if you could programmatically set the value of a file input. Yeah. Security issues.
-
DaveWalley about 10 yearsCan you click a hidden button?
-
Evan M about 9 yearsThanks! This was helpful in grabbing the actual name of the file uploaded and not the full path.
-
Dan Roberts over 8 years@emrah I think it is a convention to indicate that the variable hold a jquery object instead of a plain dom object
-
atilkan over 8 years@DanRoberts I ment where does it come from. I figured later it is weird local js var.
-
Zaker over 8 years@Thinker Is there a way to set the name of a file before it it sent to server. Thanks.
-
James111 over 8 yearsThis seems a bit over the top? The way I've listed is a lot easier and understandable.
-
rrw about 8 years@User You cannot set the filename of the file, first it would change the file being uploaded and second you cannot change input type file values.
-
Gagantous over 6 yearswhy did i get error Cannot read property 'name' of undefined?
-
Tarek.Eladly about 6 yearsthanks man i was searching for this i know this is not the subject but it was my answer you know ;)